Learn Android App Dev: Using Alert Dialog
- Amey Lokhande
- Jan 23, 2017
- 2 min read
Hello! In this post, I will give the steps on how to create an Alert Dialog box in your App. Alert Dialog is generally used when you want to show a popup message box. For ex, when you want to quit your app, so you can use Alert dialog.
So here are the steps:
1) First of all, drag a button from the widgets which we will use to show the Alert Dialog. Name the button as 'Quit?'. This Dialog will be used to quit the App gracefully.
2) Now I will explain how to write the Java code:
i) Declare the Button in your Main Activity class.
ii) Then create a ShowMessage method in your OnCreate Method. Cast your quit button in that method.
iii) Then create a SetOnClickListener method for the button which will decide the working of the Alert Dialog.

iv) In that method use Alert Builder to create a new Alert. Now follow the syntax as I have shown. I have written the comments for every line of code which will explain the code line by line.
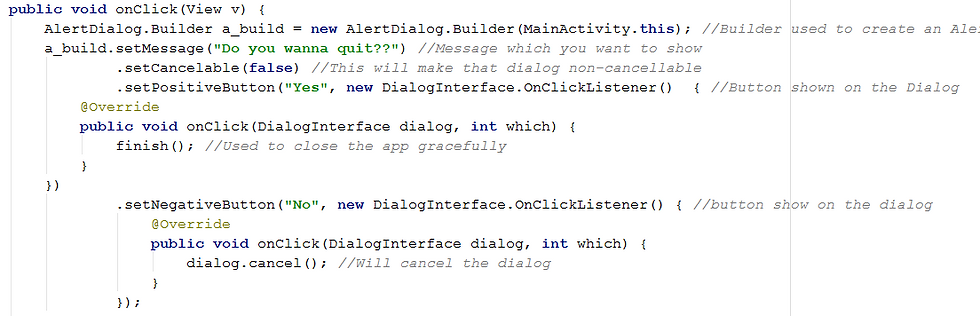
v) The above code decides the working of the Alert Dialog and will create two buttons named as Yes and No which will do the job of closing the app properly,
vi) Now create Alert using the following code:
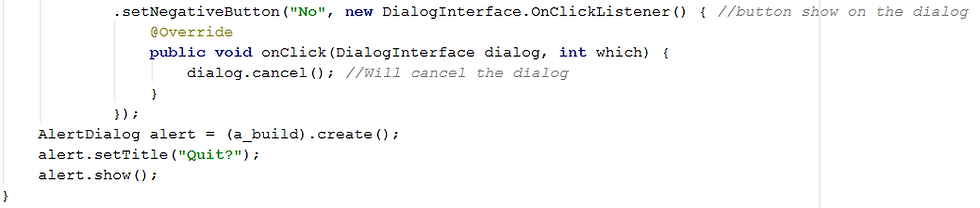
vii) The above code will set Title of the Alert Dialog to "Quit". And also the alert.show will do the job of showing the Alert Dialog.
Screenshot of the working app:
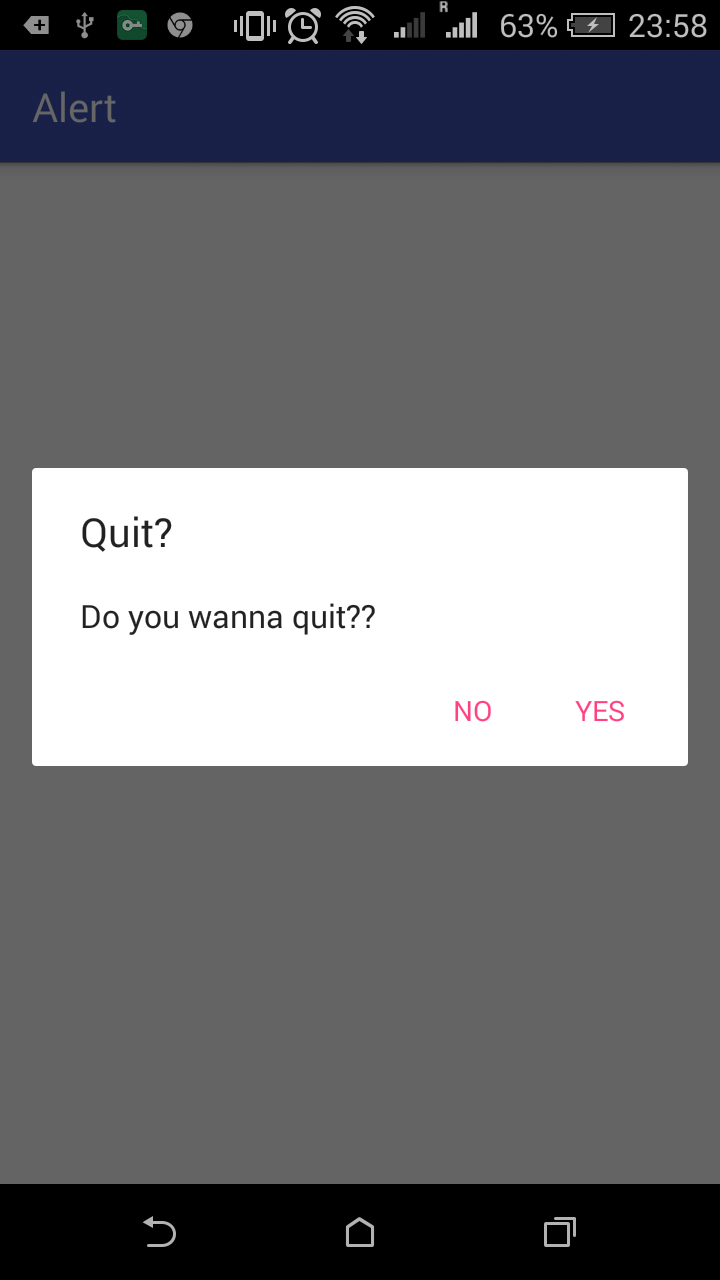
Java Code for the app:
package com.example.ameylokhande.alert;
import android.content.DialogInterface; import android.support.v7.app.AlertDialog; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button;
public class MainActivity extends AppCompatActivity { private static Button btn_quit;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ShowMessage();
} public void ShowMessage(){ btn_quit = (Button)findViewById(R.id.button);
btn_quit.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { AlertDialog.Builder a_build = new AlertDialog.Builder(MainActivity.this); //Builder used to create an Alert a_build.setMessage("Do you wanna quit??") //Message which you want to show .setCancelable(false) //This will make that dialog non-cancellable .setPositiveButton("Yes", new DialogInterface.OnClickListener() { //Button shown on the Dialog @Override public void onClick(DialogInterface dialog, int which) { finish(); //Used to close the app gracefully } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { //button show on the dialog @Override public void onClick(DialogInterface dialog, int which) { dialog.cancel(); //Will cancel the dialog } }); AlertDialog alert = (a_build).create(); alert.setTitle("Quit?"); alert.show(); } }); }
}
Thats all! Thanks!
Comments