Dictionaries in python
- Saransh Dabas
- Jul 12, 2017
- 4 min read

Dictionaries in python behave pretty much as dictionaries in real life. Whenever we want to know something about some word or value we lookup in the dictionary. A dictionary in python is a collection of key-values pairs. A key may have multiple values but each key is unique in dictionary. The elements (key-values pairs) in a dictionary are unordered, they can only be accessed using keys and not indices. If we know a key we can access the value corresponding to that key. The values can be of any data type but keys can only be of immutable data types such as numbers, strings and tuples.
Key-values pairs are clubbed together inside curly braces and (:) separate keys from values, on the left side of : we have unique key value and on the right side we can have any number of values for that key.
In this program it is shown how dictionaries are created:
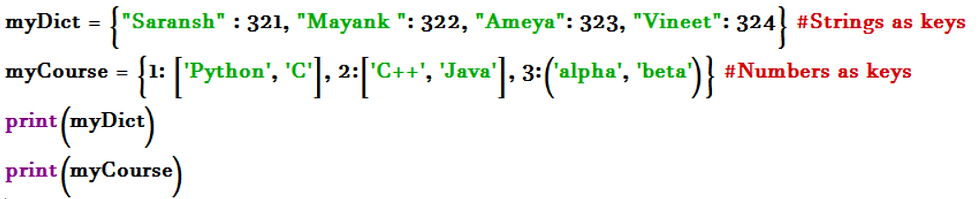
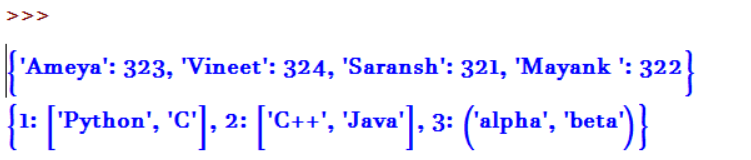
Note that the dictionary is not printed as in the same order written in the program, this is because dictionaries do not have specific order as the values are accessed using keys not indices.
i) Accessing values
We can access values in a dictionary using key values using square brackets just like lists but instead of indices we pass key value as shown in this example:
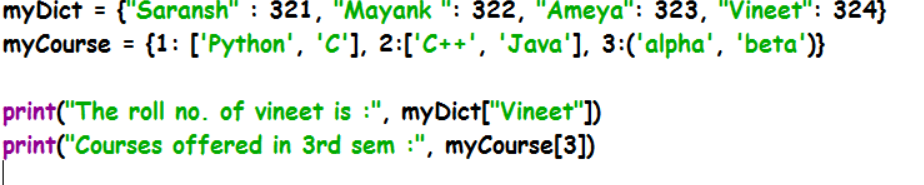

ii) Updating elements
The updation in dictionaries is very similar to that of lists, in place of indices you just need to pass the key whose value you need to update like in this example:
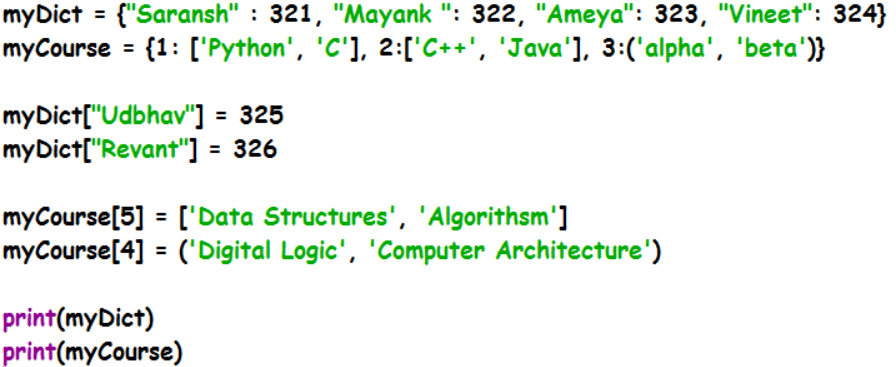

If you want to add elements of one dictionary then you can make use of update method:


iii) Deleting elements
There are three forms of deletion:
a) Deleting an element
We can delete an element from the dictionary using its key value.
b) Clear
Deletes all the elements of the dictionary making it empty and the empty dictionary is accessible.
c) Deleting the dictionary
The entire dictionary along with its elements is deleted from the memory, after deletion of the dictionary we cannot access the dictionary and we will get error if we try doing so.
All the three deletions:
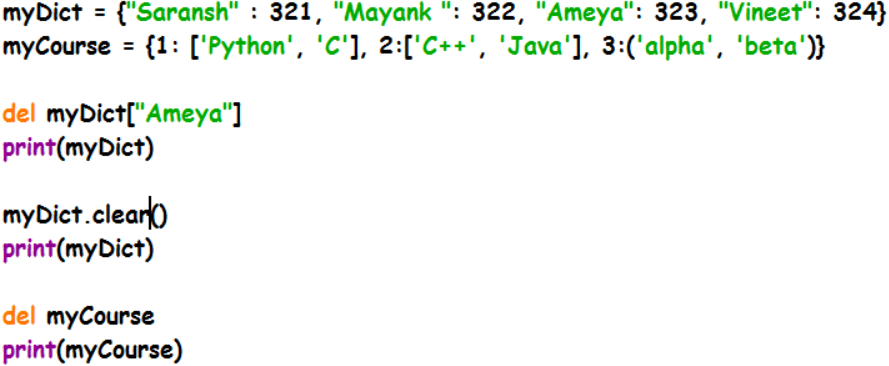
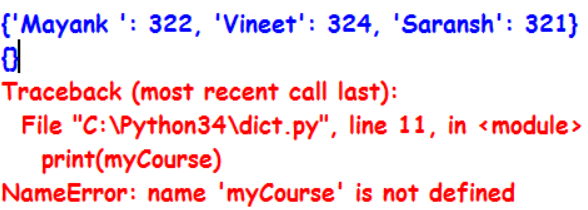
Alternatively you can use pop method to delete an element, pop method returns the value which has been deleted so if you wish to store the value in some variable as shown here:
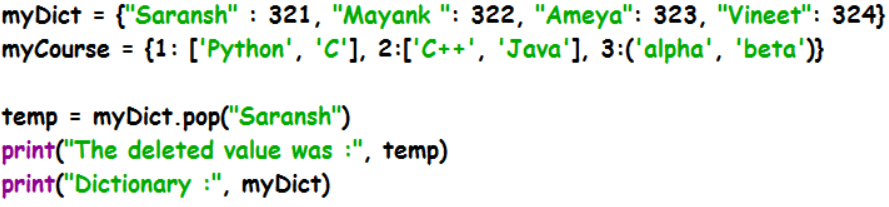

iv) Dictionary methods
a) D.items()
Returns the items in dictionary i.e list of tuples of pairs of keys and values.
b) D.keys()
Returns the list of keys in the dictionary
c) D.values() Returns the list of values in the dictionary
Example:
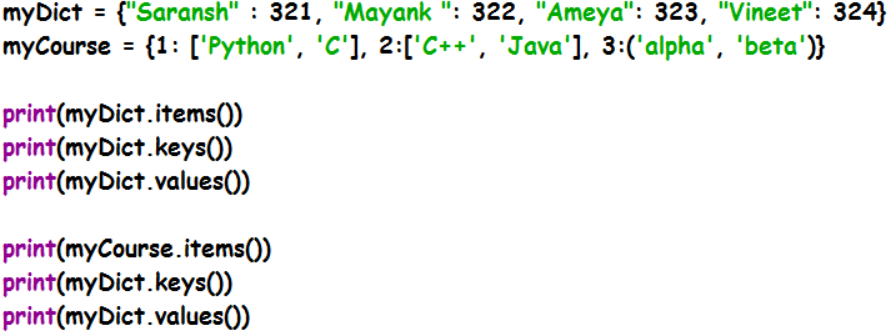

The output may not seem like a list, but it is actually and you can access values using idices.
e) D.copy()
This method returns a copy of dictionary D which can be assigned to some other variable. We cannot directly assign one dictionary to some other variable, if we do so then the new variable will actually be a reference to the same dictionary so any changes we try to make with this variable are going to be reflected in the original dictionary.


v) Dictionary functions
a) len(dict)
This function returns the total number of key, value pairs present in a dictionary dict.
b) str(dict)
Converts the dictionary into string which is printable.

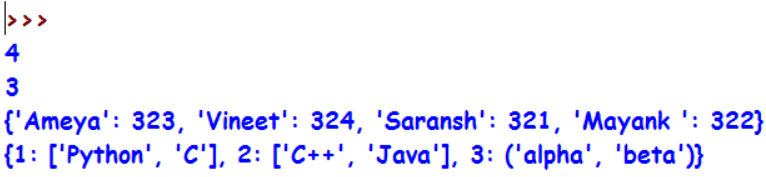
Some more ways to create a dictionary:
i) Using dict keyword
a) By assignment
b) By tuples
Examples:

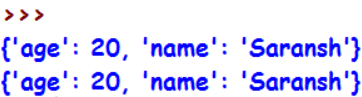
ii) Using comprehension
Just like list comprehensions we can create dictionaries in the same fashion, some of the examples are:
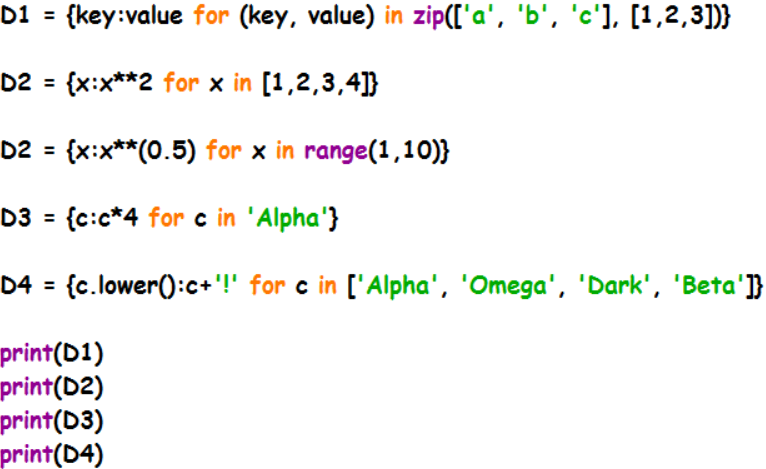

zip function is used to zip together 2 or more lists as tuples, i.e it takes two or more lists as the arguments and club together items from each list at same together and put them in tuples and returns the list of tuples.
Code section:
Creating dictionaries
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} #Strings as keys myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')} #Numbers as keys print(myDict) print(myCourse)
i) Accessing values
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')}
print("The roll no. of vineet is :", myDict["Vineet"]) print("Courses offered in 3rd sem :", myCourse[3])
ii) Updating elements: myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')} myDict["Udbhav"] = 325myDict["Revant"] = 326myCourse[5] = ['Data Structures', 'Algorithsm']myCourse[4] = ('Digital Logic', 'Computer Architecture')print(myDict)print(myCourse)
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')}
myDict.update(myCourse) print(myDict)
iii) Deleting elements
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')}
del myDict["Ameya"] print(myDict)
myDict.clear() print(myDict)
del myCourse print(myCourse)
pop()
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')}
temp = myDict.pop("Saransh") print("The deleted value was :", temp) print("Dictionary :", myDict)
iv) Dictionary methods
D.items(), D.keys(), D.values()
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')}
print(myDict.items()) print(myDict.keys()) print(myDict.values())
print(myCourse.items()) print(myDict.keys()) print(myDict.values())
D.copy()
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')}
newDict1 = myDict #Reference to myDict newDict1["Udbhav"] = 325 #This change will be reflected in myDict also print(newDict1) print(myDict)
newDict2 = myCourse.copy() #Separate copy independent of myCourse newDict2[4] = ['DLD', 'CAO'] #This change will not be reflected in myCourse print(newDict2) print(myCourse)
v) Dictionary functions
myDict = {"Saransh" : 321, "Mayank ": 322, "Ameya": 323, "Vineet": 324} myCourse = {1: ['Python', 'C'], 2:['C++', 'Java'], 3:('alpha', 'beta')}
print(len(myDict)) print(len(myCourse))
print(str(myDict)) print(str(myCourse))
Other ways to create dictionaires
i) Using dict keyword
D1 = dict(name = "Saransh", age = 20) D2 = dict([('name', 'Saransh'), ('age', 20)])
print(D1) print(D2)
ii) Comprehensions
D1 = {key:value for (key, value) in zip(['a', 'b', 'c'], [1,2,3])}
D2 = {x:x**2 for x in [1,2,3,4]}
D2 = {x:x**(0.5) for x in range(1,10)}
D3 = {c:c*4 for c in 'Alpha'}
D4 = {c.lower():c+'!' for c in ['Alpha', 'Omega', 'Dark', 'Beta']}
print(D1) print(D2) print(D3) print(D4)
Comments