Lists in python

A list is a data structure in python which act as a collection of elements. The special thing about python list is that they can contain elements of different data types.
i) Creating lists
The simplest way to create a list to give comma separated values inside square brackets, a list can also be initialized empty with just square brackets as shown in the following program.


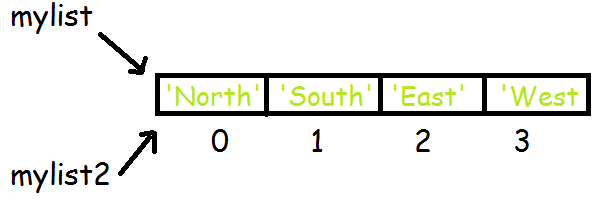
There is one more important point I would like to discuss about python lists, when a python list is created the variable name of the list is nothing but a reference to a list so let us say we created a list and given it a name myList and we tried to copy the string to some other variable name using assignment (=) operator myList2 then what happens is that both myList and myList2 will point to same memory location and any change made to myList2 will be reflected in myList too as shown in the following example:
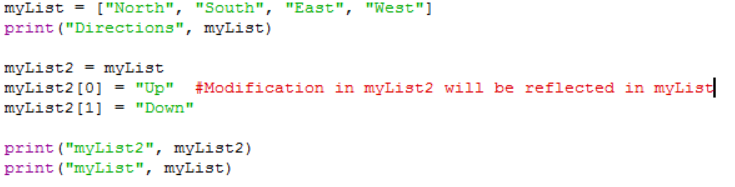

To overcome this problem we have several methods:
Using myList2 = myList[:] and
We also have something called copy method which create a separate copy of the list but it is present in the copy module which we need to import first.


Both of the above two methods provide us a shallow copy of the list, we will see more about deep copy and shallow copy later in object oriented programming with python tutorial.
Another way of creating list is using list() method which can take argument of various types as shown in the following example:


ii) Accessing values:
Just like strings here also indexing, slicing rules work, the index of the starting element is 0. Here is a program to show how indexing and slicing works:
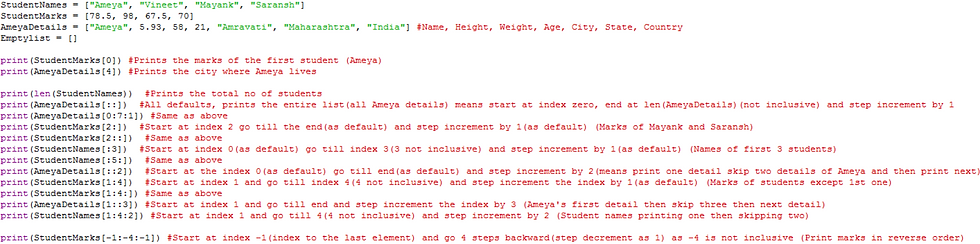

iii) Updating lists:
We can update lists using by assigning some value at particular index, it is a kind of replacement, we will see more about updating lists in subsequent sections:
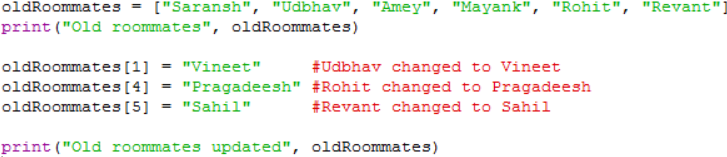

iv) Deleting:
We can delete an element from the list using del method if we know the index of the value we want to delete, here is how


Syntax for del method is del(myList[index]), you have to be careful while using multiple del statements because each time you delete an element the size of list decreases by 1 hence the element that would be present at some location it's index may get decreased or it may remain same depending whether it is present on the left or the right side of the deleted element.
It may be possible that because the list size is decreased after applying del method we try to access a value which is no longer available at that index, as shown in this example:


v) Basic operations on lists
i) len()
Used to get the length of the string
ii) Concatenation
Two or more lists can be concatenated (Means that the elements from the second lists are attached to the end of the first) and the second list remains unchanged.
iii) Repetition
We can use * on list to repeat some elements
iv) Membership
We can check for elements if they are present in a list or not.
Here is the code showing the above operations:
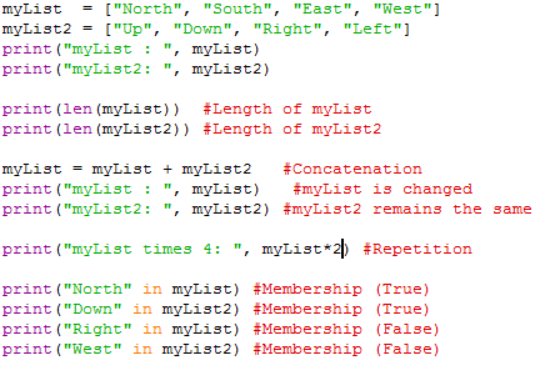

Some useful built-in list functions and methods:
i) len(), max(), min()
These methods take a list as an argument and returns the length, maximum value and minimum value respectively of the list. Following is a program showing how these functions gives the output.
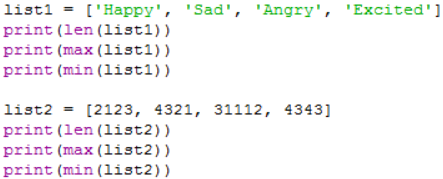
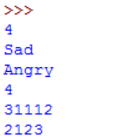
Note that comparison between two strings is done lexicographically that is:
First the first two items are compared, and if they differ this determines the outcome of the comparison.
If they are equal, the next two items are compared, and so on, until either sequence is exhausted.
If we try to get a minimum or maximum value in case a list has dissimilar data type we might get some error.


The cause of error is that we cannot compare datatypes int with str but in case we have float and integers this will work.
ii) append()
Using append we can insert a value at the back of the list, the value can be a single one or an iterable like list, here comes the program:
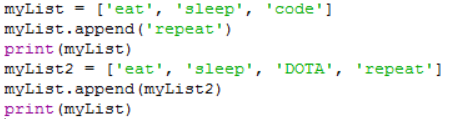

iii) sort() and sorted()
Sort method helps to sort the elements of lists in ascending order, the elements should be of same type inside the list, one obvious exception is float and int, in case of string values the list is sorted lexicographically.
We can have two argument called as key and reverse, key specifies what should be the criteria for sorting and reverse = True says that after sorting we want to reverse the list.
The only difference between sort() and sorted() is that sorted() takes list as an argument hence we can assign sorted list to some other variable name and original list remains the same while sort() is a method called on a list itself and so the original list gets modified as shown here:
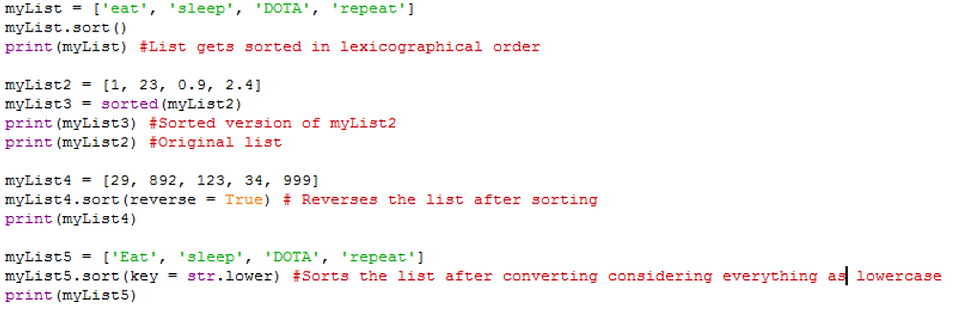

iv) extend()
This method perform the same functionality as concatenation as shown:


v) reverse() and reversed()
This method is used to reverse a list:


reversed() method helps to reverse the list and assign it to some other variable name, also reversed() method returns an iterator object and so we need to convert it to a list to get a list out of that object:


vi) index() and count()
index() method takes a value as an argument and returns the index of that value in the list while count simply returns the number of times an element occurred in a list passed as an argument:


vii) insert(), remove() and pop()
insert() method takes two argument first one is the index where you want to insert the value in the list and second one is the value
remove() method takes the element you want to remove as an argument
pop() method takes index of the element you want to remove and returns the element after removal, if nothing is specified then it removes and returns the last element.
One major difference between pop() and remove() is that pop() returns the value it removes while remove doesn't, it returns nothing.
remove() deletes the first occurrence of the element.
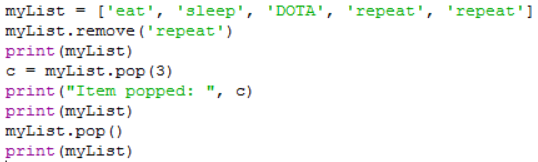
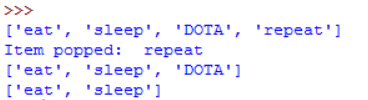
Insertion, Deletion and Replacement:
We can use slicing to perform insertion, deletion and replacement in a list as shown in this example:
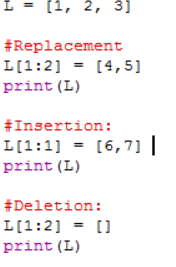

1:2 slice is equivalent to saying that start at one index and go till 2(not inclusive) hence it means that we are getting effectively only index 1 where we insert 4 and 5
1:1 slice is equivalent to saying that start at one index and go till 1(not inclusive) hence effectively we are starting at one and getting nothing further hence 6 and 7 gets inserted after index at index 1.
Deletion is another kind of replacement, the value that replaces here is empty hence we effectively deletes the element from the list.
We can also delete values using del(), it takes a slice of list as an argument or a single element and removes it as shown:
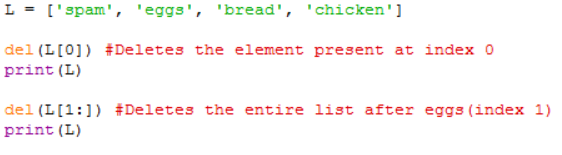

List comprehensions
There is elegant way of combining for loops and lists to write codes in single line which would otherwise have taken more no. of lines as shown in this example:


Map
Applies a function to all the items in a list and collects the result in a new list just like mathematical mapping:

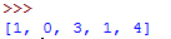
So that was all guys for lists, I tried covering most of the methods available and we will use them frequently to write beautiful programs.
Code section:
Assigning one list to another list using name problem:
myList = ["North", "South", "East", "West"] print("Directions", myList)
myList2 = myList[:] myList2[0] = "Up" #Modification in myList2 will be reflected in myList myList2[1] = "Down"
print("myList2", myList2) print("myList", myList)
Problem solutions:
import copy myList = ["North", "South", "East", "West"] print("Directions", myList)
myList2 = myList[:] myList3 = copy.copy(myList2)
myList2[0] = "Up" #Modification in myList2 will not be reflected in myList as it is a copy of myList myList2[1] = "Down"
myList3[2] = "Right" #Modification in myList3 will not be reflected in myList as it is a copy of myList myList3[3] = "Left"
print("myList2", myList2) print("myList3", myList3) print("myList", myList)
i) Creating lists:
StudentNames = ["Ameya", "Vineet", "Mayank", "Saransh"] StudentMarks = [78.5, 98, 67.5, 70] AmeyaDetails = ["Ameya", 5.93, 58, 21, "Amravati"] #Name, Height, Weight, Age, City Emptylist = []
print(StudentNames, StudentMarks, AmeyaDetails, Emptylist, sep='\n')
using list() method
List1 = list("Saransh Dabas") #String as argument List2 = list(range(1,66,2)) #List of a range of values List3 = list((1,242,2,123,"Hi","There",2.3,23,44)) #Tuples as an argument List4 = list({1:"Revant", 2:"Sahil", 3:"Shyam", 4:"Ram"}) #Dictionary as data items
print(List1, List2, List3, List4, sep='\n')
ii) Accessing list values
StudentNames = ["Ameya", "Vineet", "Mayank", "Saransh"] StudentMarks = [78.5, 98, 67.5, 70] AmeyaDetails = ["Ameya", 5.93, 58, 21, "Amravati", "Maharashtra", "India"] #Name, Height, Weight, Age, City, State, Country Emptylist = []
print(StudentMarks[0]) #Prints the marks of the first student (Ameya) print(AmeyaDetails[4]) #Prints the city where Ameya lives
print(len(StudentNames)) #Prints the total no of students print(AmeyaDetails[::]) #All defaults, prints the entire list(all Ameya details) means start at index zero, end at len(AmeyaDetails)(not inclusive) and step increment by 1 print(AmeyaDetails[0:7:1]) #Same as above print(StudentMarks[2:]) #Start at index 2 go till the end(as default) and step increment by 1(as default) (Marks of Mayank and Saransh) print(StudentMarks[2::]) #Same as above print(StudentNames[:3]) #Start at index 0(as default) go till index 3(3 not inclusive) and step increment by 1(as default) (Names of first 3 students) print(StudentNames[:5:]) #Same as above print(AmeyaDetails[::2]) #Start at the index 0(as default) go till end(as default) and step increment by 2(means print one detail skip two details of Ameya and then print next) print(StudentMarks[1:4]) #Start at index 1 and go till index 4(4 not inclusive) and step increment the index by 1(as default) (Marks of students except 1st one) print(StudentMarks[1:4:]) #Same as above print(AmeyaDetails[1::3]) #Start at index 1 and go till end and step increment the index by 3 (Ameya's first detail then skip three then next detail) print(StudentNames[1:4:2]) #Start at index 1 and go till 4(4 not inclusive) and step increment by 2 (Student names printing one then skipping two)
print(StudentMarks[-1:-4:-1]) #Start at index -1(index to the last element) and go 4 steps backward(step decrement as 1) as -4 is not inclusive (Print marks in reverse order)
iii) Updating lists:
oldRoommates = ["Saransh", "Udbhav", "Amey", "Mayank", "Rohit", "Revant"] print("Old roommates", oldRoommates)
oldRoommates[1] = "Vineet" #Udbhav changed to Vineet oldRoommates[4] = "Pragadeesh" #Rohit changed to Pragadeesh oldRoommates[5] = "Sahil" #Revant changed to Sahil
print("Old roommates updated", oldRoommates)
iv) Deleting:
oldRoommates = ["Saransh", "Udbhav", "Amey", "Mayank", "Rohit", "Revant"] print("Old roommates", oldRoommates)
del(oldRoommates[1]) #Udbhav is removed
print("Old roommates in new room", oldRoommates)
oldRoommates = ["Saransh", "Udbhav", "Amey", "Mayank", "Rohit", "Revant"] print("Old roommates", oldRoommates)
del(oldRoommates[1]) #Udbhav is removed del(oldRoommates[4]) #Trying to remove Rohit but he has been shifted one place hence Revant will get removed
print("Old roommates in new room", oldRoommates)
v) Basic operations on lists:
myList = ["North", "South", "East", "West"] myList2 = ["Up", "Down", "Right", "Left"] print("myList : ", myList) print("myList2: ", myList2)
myList = myList + myList2 #Concatenation print("myList : ", myList) #myList is changed print("myList2: ", myList2) #myList2 remains the same
print("myList times 4: ", myList*2) #Repetition
print("North" in myList) #Membership (True) print("Down" in myList2) #Membership (True) print("Right" in myList) #Membership (False) print("West" in myList2) #Membership (False)
v) len(), min() and max()
list1 = ['Happy', 'Sad', 'Angry', 'Excited'] print(len(list1)) print(max(list1)) print(min(list1))
list2 = [2123, 4321, 31112, 4343] print(len(list2)) print(max(list2)) print(min(list2))
v) append()
myList = ['eat', 'sleep', 'code'] myList.append('repeat') print(myList) myList2 = ['eat', 'sleep', 'DOTA', 'repeat'] myList.append(myList2) print(myList)
vi) sort() and sorted()
myList = ['eat', 'sleep', 'DOTA', 'repeat'] myList.sort() print(myList) #List gets sorted in lexicographical order
myList2 = [1, 23, 0.9, 2.4] myList3 = sorted(myList2) print(myList3) #Sorted version of myList2 print(myList2) #Original list
myList4 = [29, 892, 123, 34, 999] myList4.sort(reverse = True) # Reverses the list after sorting print(myList4)
myList5 = ['Eat', 'sleep', 'DOTA', 'repeat'] myList5.sort(key = str.lower) #Sorts the list after converting considering everything as lowercase print(myList5)
vi) extend()
myList = ['eat', 'sleep', 'DOTA', 'repeat']
myList2 = ['eat', 'sleep', 'DBS', 'repeat']
myList.extend(myList2) print(myList)
vii) reverse() and reversed()
myList = ['eat', 'sleep', 'DOTA', 'repeat'] myList.reverse() print(myList)
myList = ['eat', 'sleep', 'DOTA', 'repeat'] myList2 = list(reversed(myList)) print(myList) print(myList2)
vii) index() and count()
myList = ['eat', 'sleep', 'DOTA', 'repeat', 'repeat'] print(myList.index('DOTA')) #What is the index of 'DOTA' print(myList.count('repeat')) #How many times 'repeate' has occurred
viii) insert(), remove() and pop()
myList = ['eat', 'sleep', 'DOTA', 'repeat', 'repeat'] myList.remove('repeat') print(myList) c = myList.pop(3) print("Item popped: ", c) print(myList) myList.pop() print(myList)
ix) Insertion, Deletion and Replacement:
L = [1, 2, 3]
#Replacement L[1:2] = [4,5] print(L)
#Insertion: L[1:1] = [6,7] print(L)
#Deletion: L[1:2] = [] print(L)
L = ['spam', 'eggs', 'bread', 'chicken']
del(L[0]) #Deletes the element present at index 0 print(L)
del(L[1:]) #Deletes the entire list after eggs(index 1) print(L)
x) List comprehensions:
myList = [alphabet*4 for alphabet in 'Saransh'] #Take each alphabet or character in 'Saransh', repeate it 4 times and append it to the list print(myList)
xi) Map
List = list(map(abs, [-1,0,-3,1,4])) print(List)