Strings in python
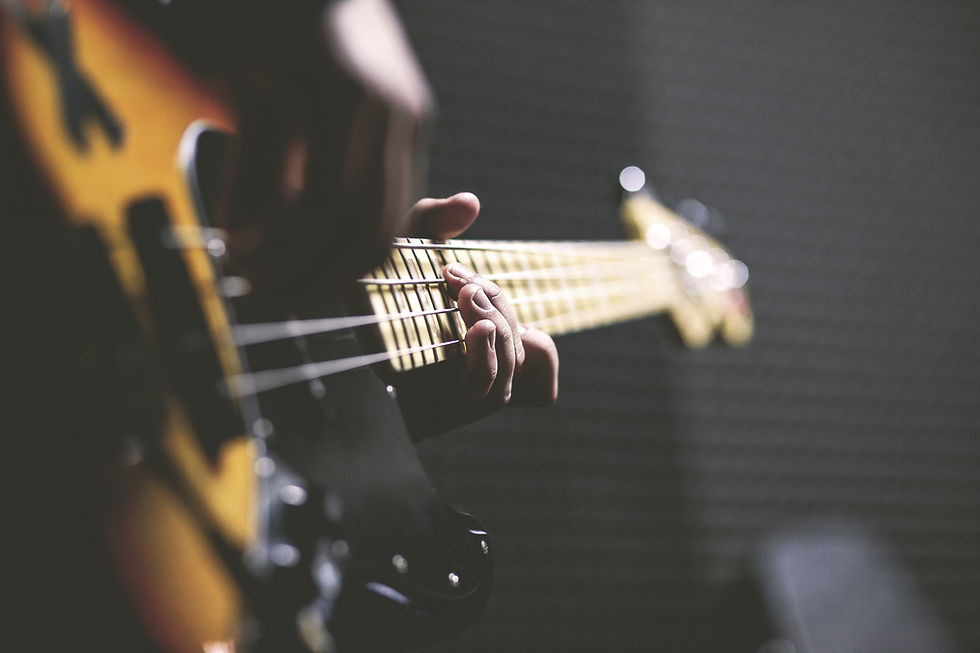
Strings are nothing but a sequence of characters, in python we don't have any datatype for character, we simply have strings and a character is treated as a string of one length. A string is created simply by giving some value inside single, double or triples quotes as shown in this example:
Since strings are made up of units of character it is also called as compound data type.
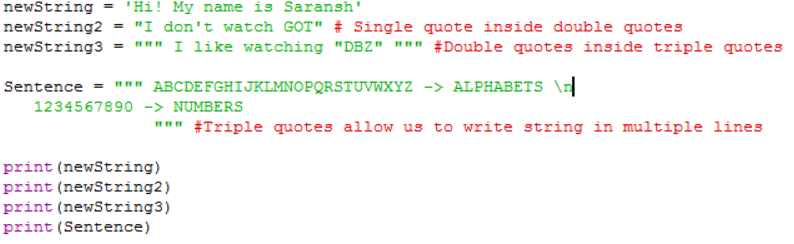
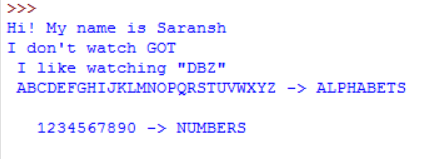
We can use double quotes in case we want single quotes in our string itself, also if we want double quotes in our string we can go with triple quotes and also using triple quotes we can write a string in multiple lines in our editor as shown in the above program.
Strings are immutable:
Strings are immutable means that once they are created as a unit of characters, the characters in it cannot be changed i.e we cannot do something like:

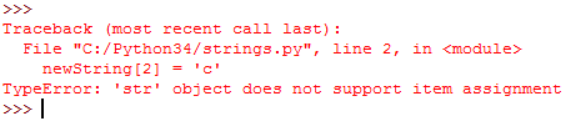
String operations
String operations are the operations which can be performed on a string, these are:
i) String concatenation
By concatenation we mean taking two strings and merging them. This is done using + operator.


ii) Repetition
A string can be multiplied with a constant to concatenate with itself multiple times.
We can say that newString*3 is equivalent to newString + newString + newString.


iii) Indexing
We can access a particular element from the string using indices. Indices are nothing but an integer value given for each position in the string, they start with 0, i.e the first character has the index as 0 and second one as 1 and so on, the index of last element is length of string-1. Python also supports negative indices where -1 is the index of the last element, -2 is the index of second last element and so on.

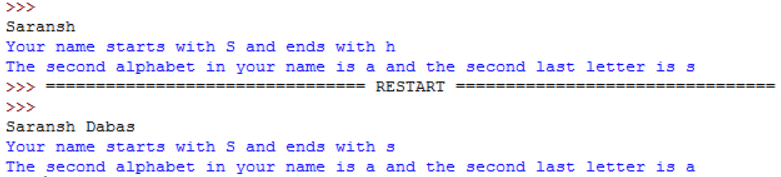
iii) Slicing
We can access a slice from the string i.e a sub-string of the string, slicing is just an extension to indexing, inside brackets we can pass more values separated by : (ex- myString[i:j:k]) to perform the functionalities as shown below:
i -> starting index for the substring you want to generate, if not specified default value is taken as 0.
j -> ending index for the substring, if not specified default value is taken as len(myString)
k -> step increment/ decrement for the substring values, by default it is taken as 1.
The permissible values for i,j and k are only integers i.e we cannot do something like myString[1.2:2.3:4.5].
The following program depicts the effects of all the possible combinations of i, j and k:

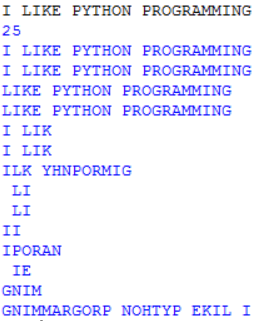
Note that the index of the last element is len(myString)-1 not len(myString) because len(myString) returns the actual length of the string which is one more than the last index as indices start at 0.
We can check whether a number or string is palindrome or not by using the reversing technique as shown below:
A palindrome is a number or word which is same if written backwards as written forward


iv) Escape sequences
We are mainly going to focus on two escape sequences \t and \n, if we need more than these I will introduce them there itself. \t is used to give a space of 4 spaces or 1 tab and \n stands for newline i.e the content after this will be displayed in the next line as shown in the following program:


v) For loop to access the characters in a given string:
A string is a iterable so we can access its values using indices or by using for loop as shown here:
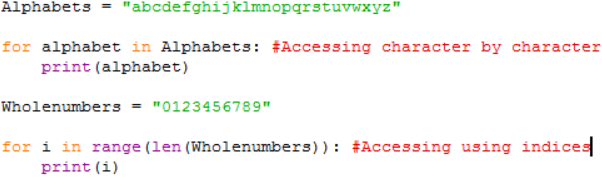

vi) Membership
We can check whether a character or a substring is present in the string or not using in keyword, also we can check for a character not to be present using not in keyword as shown in this program:

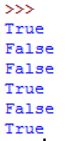
Some useful string methods:
i) count()
Count the number of occurrence of a character or a sub-string in a string
ii) ASCII <-> Char
We can convert a character to its ASCII value using ord() and ASCII value to character value using chr()
iii) replace()
This method takes 2 arguments, first one is the character or the sub-string you want to replace and second one is the character or string you want to replace it with.
iv) lower()
Converts all the characters in a string to lowercase.
v) upper()
Converts all the characters in a string to uppercase.
vi) find()
Finds the first occurrence of the character or sub-string and returns the index value.
vii) isalpha()
Checks whether all the characters in a given string are alphabets or not.
viii) isdigit()
Checks whether all the characters in a given string are digits or not.
ix) isupper()
Checks whether all the characters in a given string are uppercase or not.
x) islower()
Checks whether all the characters in a given string are uppercase or not.
xi) istitle()
Checks if all the space separated words in a string are in Tile case or not.
xi) endswith()
Checks whether a string ends with a particular character or sub-string or not.
xii) startswith()
Checks whether a string starts with a particular character or sub-string or not.
Above points will be get cleared from this program:
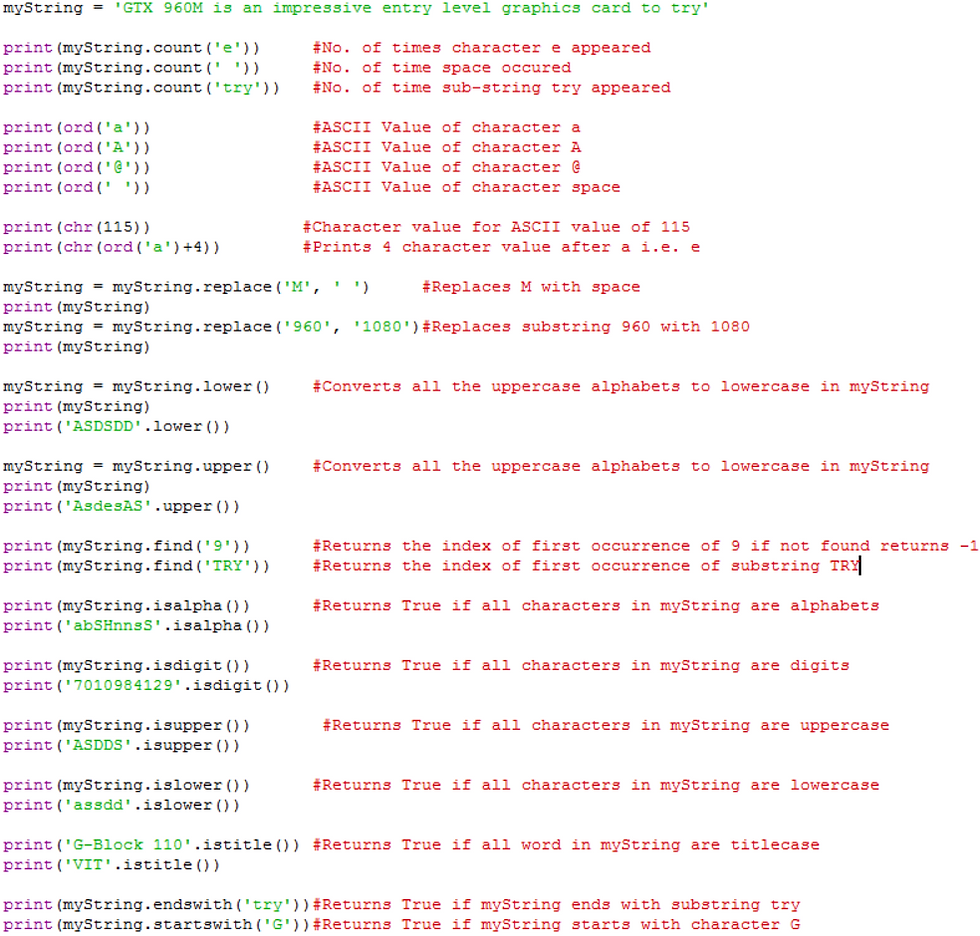
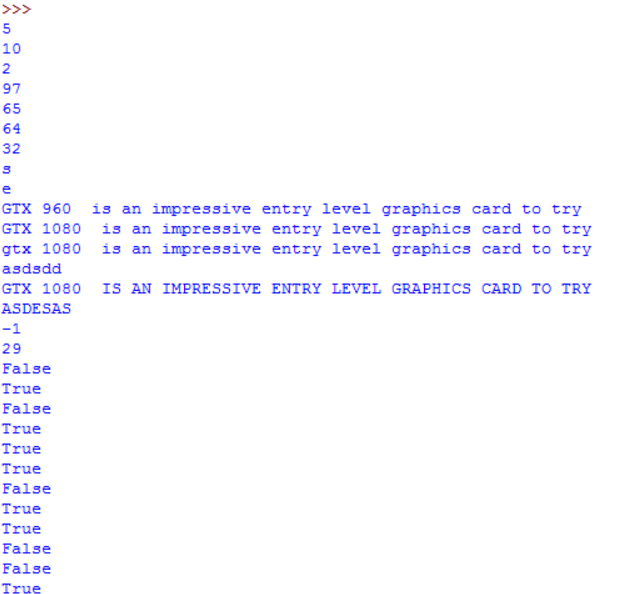
As this tutorial is already going too long, I will discuss string formatting in other one, thanks for reading this, see you in the next one.
Code Section:
i) Single, double, triple quotes:
newString = 'Hi! My name is Saransh' newString2 = "I don't watch GOT" # Single quote inside double quotes newString3 = """ I like watching "DBZ" """ #Double quotes inside triple quotes
Sentence = """ ABCDEFGHIJKLMNOPQRSTUVWXYZ -> ALPHABETS \n 1234567890 -> NUMBERS """ #Triple quotes allow us to write string in multiple lines
print(newString) print(newString2) print(newString3) print(Sentence)
ii) String assignment, incorrect program:
newString = 'Alpha-Omega' newString[2] = 'c' print(newString)
iii) String concatenation
hobby = "He is a very good football player" passion = " and he likes programming"
print(hobby + passion)
iv) Repetition
troubleMessage = " We are in danger! I repeat "
print(troubleMessage*3) #Take string troubleMessage and perform conctenation 2 times print(troubleMessage+troubleMessage+troubleMessage)
v) Indexing
name = str(input())
print("Your name starts with {} and ends with {}".format(name[0], name[-1])) print("The second alphabet in your name is {} and the second last letter is {}".format(name[1], name[-2]))
vi) Slicing
myString = str(input())
k = len(myString) print(len(myString)) #Prints the length of myString print(myString[::]) #All defaults, prints the entire string means start at index zero, end at len(myString)(not inclusive) and step increment by 1 print(myString[0:k:1]) #Same as above print(myString[2:]) #Start at index 2 go till the end(as default) and step increment by 1(as default) print(myString[2::]) #Same as above print(myString[:5]) #Start at index 0(as default) go till index 5(5 not inclusive) and ste[ increment by 1(as default) print(myString[:5:]) #Same as above print(myString[::2]) #Start at the index 0(as default) go till end(as default) and step increment by 2(means print one character skip two characters) print(myString[1:4]) #Start at index 1 and go till index 4(4 not inclusive) and step increment the index by 1(as default) print(myString[1:4:]) #Same as above print(myString[:9:3]) #Start at index 0(as default) and go till index 9(9 not inclusive) and step increment the index by 3 print(myString[3::4]) #Start at index 3 and go till end(as default) ans step increment by 4 print(myString[1:6:2]) #Start at index 1 and go till 6(6 not inclusive) and step increment by 2
print(myString[-1:-5:-1]) #Start at index -1(index to the last element) and go 4 steps backward(step decrement as 1) as -5 is not inclusive print(myString[-1:-k-1:-1]) #Reverses the string, start from backward and go till first with step decrement as 1
vii) Palindrome string checking
myString = str(input())
k = len(myString)
revmyString = myString[-1:-k-1:-1] #Reverses the string, start from backward and go till first with step decrement as 1
if myString == revmyString: print("{} is palindrome".format(myString))
viii) Escape sequences
mobilePrices = "Nokia\t6000\nApple\t55000\nXiomi\t3000" print(mobilePrices)
ix) For loop access
Alphabets = "abcdefghijklmnopqrstuvwxyz"
for alphabet in Alphabets: #Accessing character by character print(alphabet)
Wholenumbers = "0123456789"
for i in range(len(Wholenumbers)): #Accessing using indices print(i)
x) Membership
Alphabets = "abcdefghijklmnopqrstuvwxyz"
print('z' in Alphabets) #Returns true if the character z is present in Alphabets otherwise false print('@' in Alphabets) #Returns true if the character @ is present in Alphabets otherwise false print('w' not in Alphabets) #Returns true if the character w is not present in Alphabets otherwise false print('def' in Alphabets) #Return true if the substring def is present in Alphabets otherwise false print('qws' in Alphabets) #Return true if the substring qws is present in Alphabets otherwise false print('rts' not in Alphabets) #Return true if the substring rts is not present in Alphabets otherwise false
xi) String methods
myString = 'GTX 960M is an impressive entry level graphics card to try'
print(myString.count('e')) #No. of times character e appeared print(myString.count(' ')) #No. of time space occured print(myString.count('try')) #No. of time sub-string try appeared
print(ord('a')) #ASCII Value of character a print(ord('A')) #ASCII Value of character A print(ord('@')) #ASCII Value of character @ print(ord(' ')) #ASCII Value of character space
print(chr(115)) #Character value for ASCII value of 115 print(chr(ord('a')+4)) #Prints 4 character value after a i.e. e
myString = myString.replace('M', ' ') #Replaces M with space print(myString) myString = myString.replace('960', '1080')#Replaces substring 960 with 1080 print(myString)
myString = myString.lower() #Converts all the uppercase alphabets to lowercase in myString print(myString) print('ASDSDD'.lower())
myString = myString.upper() #Converts all the uppercase alphabets to lowercase in myString print(myString) print('AsdesAS'.upper())
print(myString.find('9')) #Returns the index of first occurrence of 9 if not found returns -1 print(myString.find('TRY')) #Returns the index of first occurrence of substring TRY
print(myString.isalpha()) #Returns True if all characters in myString are alphabets print('abSHnnsS'.isalpha())
print(myString.isdigit()) #Returns True if all characters in myString are digits print('7010984129'.isdigit())
print(myString.isupper()) #Returns True if all characters in myString are uppercase print('ASDDS'.isupper())
print(myString.islower()) #Returns True if all characters in myString are lowercase print('assdd'.islower())
print('G-Block 110'.istitle()) #Returns True if all word in myString are titlecase print('VIT'.istitle())
print(myString.endswith('try'))#Returns True if myString ends with substring try print(myString.startswith('G'))#Returns True if myString starts with character G