Loops: for loop in python
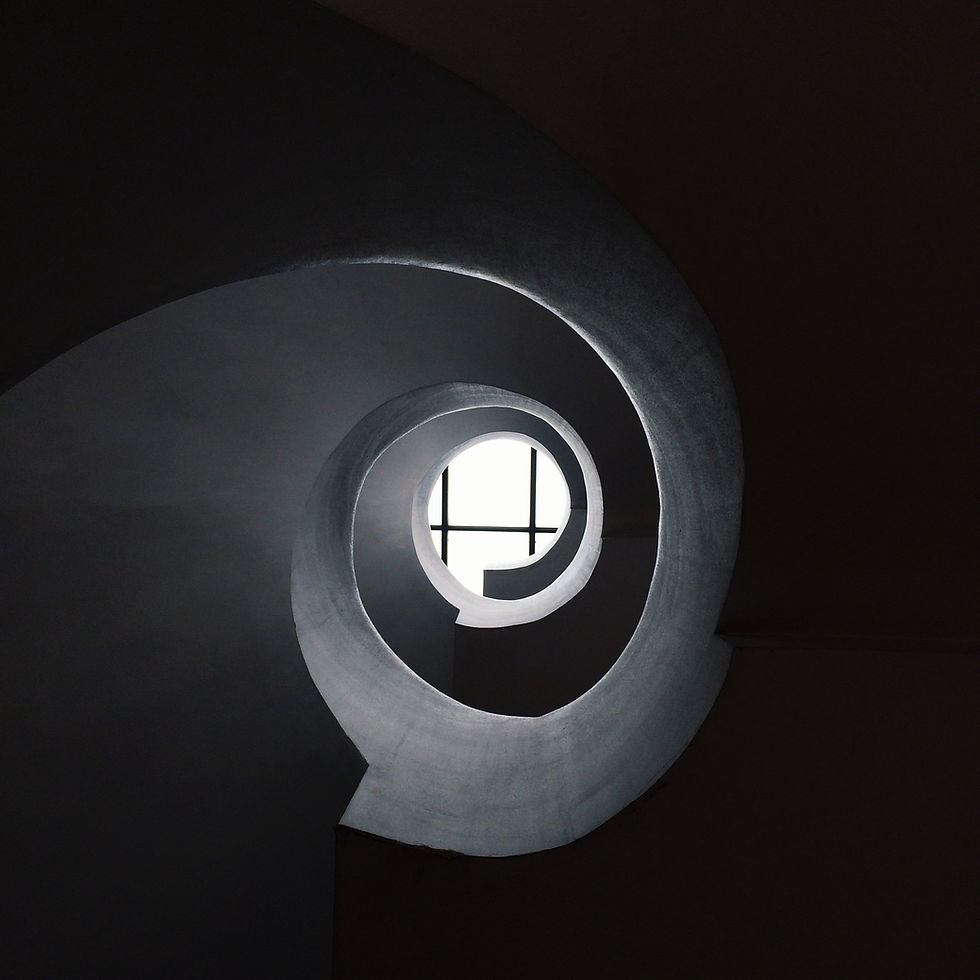
For loop is similar to while but for loop takes care of the condition also i.e unlike while loop we need not take care of the condition to make it false, the very definition of for loop specifies the how it is going to terminate. So let's see the syntax of for loop:
for iteratingVariable in Sequence:
#Conditional code
Note there are three parts of a for loop:
i) Iterating variable
The variable which is going to iterate(traverse) over the sequence is called as iterating variable
ii) Sequence
It is the sequence of numbers, or a list or tuple or string etc. basically any iterable data type.
iii) Conditional code
This is the sequence of statements you want to execute in the loop until we reach the end of the iterable i.e entire sequence gets finished.
Here is the control flow graph for for loop:
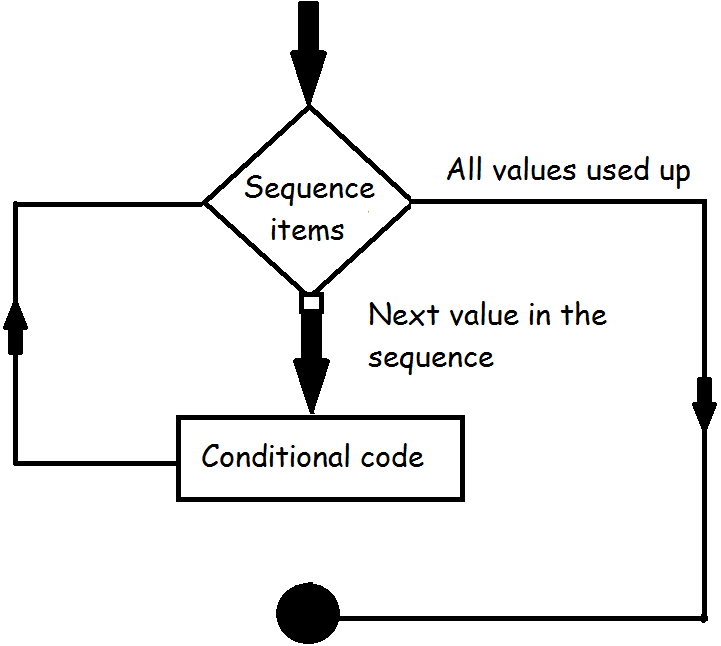
Now let's understand the for loop using one example:
One more thing before we go ahead, there is a built in function in python called range which is a sequence generator and can be used as a sequence of values in for loop, it takes three arguments, first and second arguments specifies the range of values and third one specifies the step by which the value must be incremented or decremented as shown:
sep = '\n' is used to give a space of new line between two lists.
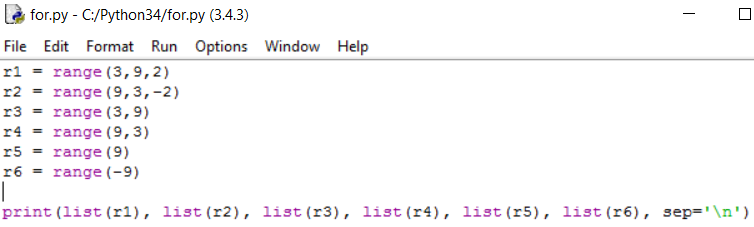
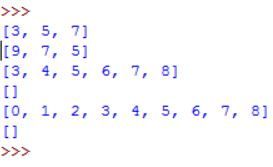
Note that the second argument value is not included in the range hence it is exclusive whereas first argument value is included hence it is inclusive.
Also if third argument by default takes the value as 1 if not specified and first argument takes the value as 0 by default if not specified.
r1 is saying that range starts from 3(including 3) and goes till 9(excluding 9) and step incremented by 2.
r2 is saying that range starts from 9(including 9) and goes till 3(excluding 3) and step decremented by 2.
r3 is saying that range starts from 3(including 3) and goes till 9(excluding 9) and by default step incremented by 1.
r4 is saying that range starts from 9 and goes till 3 and by default step incremented by 1 as we cannot reach 3 from 9 with incrementation by 1 hence we get an empty sequence, if we give such a sequence in for loop, it will not execute the statements inside it even once, such a piece of code is called as dead code.
r5 is saying that by default range starts from 0(including 0) and go till 9(excluding 9) and by default step incremented by 1.
r6 is saying that by default range starts from 0 and goes upto -9, by step increment of 1 which is obviously not possible and so we get an empty sequence.
Here, list is a data type to hold the multiple values of the sequence generated by range.
Now here is a program to print squares of n natural numbers using for loop and range:


Now for loop is not limited to iterate over some numbers, we can basically iterate over strings, lists, dictionaries etc.
ITERATING OVER A STRING:
String is basically a sequence of characters so it is a sequence which can be iterated as shown in the following program:
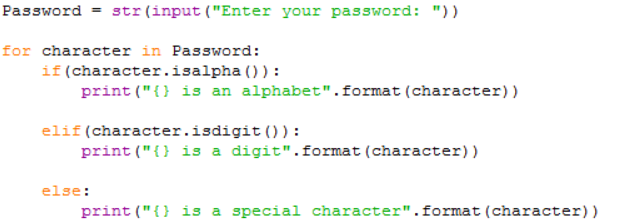
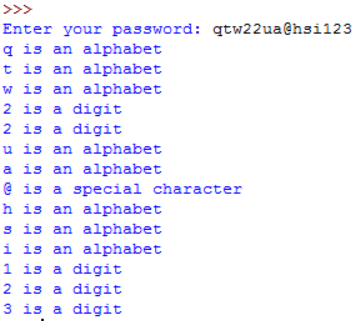
We are taking each character from the input and checking whether that character is an alphabet, a number or a special character.
ITERATING OVER A LIST AND TAKING MULTIPLE INPUTS:
We can iterate over a list of elements one by one using for loop, for example: Imagine your mom asked you to bring some vegetables 1Kg each and you are confused in remembering them, I personally gets confused in pudina and dhaniya, anyway you can write a program and prepare a list of vegetables and then you can iterate over that list later so you need not remember the vegetable names, here is how such program will look like:
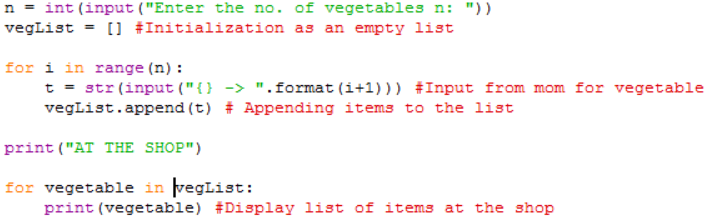

Iterating over a tuple or a set is also the same.
ITERATING OVER A DICTIONARY
Suppose your mom wants to bring you vegetables but this time each one in different quantity, so in such cases we can use dictionaries with key as vegetable and value as quantities. Here is a program showing how this works:
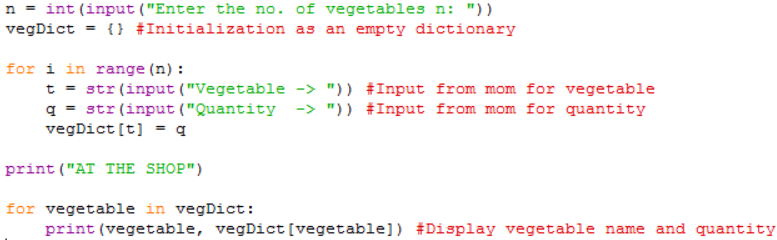
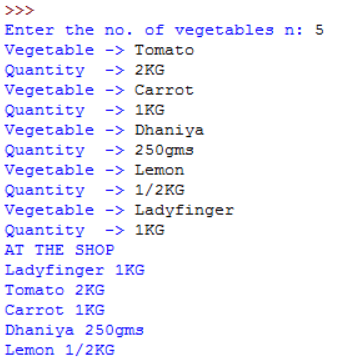
Note: If you are new to lists, strings and dictionaries and if you don't understand the above programs, do check out lists, strings, tuples, sets and dictionaries in separate tutorials.
So that's all for for loop, we will learn more about it and how it can be used to write beautiful programs in this series of tutorials, hope you liked the article, see you in the next one.
Code section:
i) Range
r1 = range(3,9,2) r2 = range(9,3,-2) r3 = range(3,9) r4 = range(9,3) r5 = range(9) r6 = range(-9)
print(list(r1), list(r2), list(r3), list(r4), list(r5), list(r6), sep='\n')
ii) Squares of n natural numbers
n = int(input("Enter the value of n: "))
for i in range(1,n+1): #natural numbers start from 1. print("{} squared equals {}".format(i,i**2))
iii) Password checking
Password = str(input("Enter your password: "))
for character in Password: if(character.isalpha()): print("{} is an alphabet".format(character))
elif(character.isdigit()): print("{} is a digit".format(character))
else: print("{} is a special character".format(character)) iv) Vegetable program using list
n = int(input("Enter the no. of vegetables n: ")) vegList = [] #Initialization as an empty list
for i in range(n): t = str(input("{} -> ".format(i+1))) #Input from mom for vegetable vegList.append(t) # Appending items to the list
print("AT THE SHOP")
for vegetable in vegList: print(vegtable) #Display list of items at the shop
v) Vegetable program using dictionary
n = int(input("Enter the no. of vegetables n: ")) vegDict = {} #Initialization as an empty dictionary
for i in range(n): t = str(input("Vegetable -> ")) #Input from mom for vegetable q = str(input("Quantity -> ")) #Input from mom for quantity vegDict[t] = q
print("AT THE SHOP")
for vegetable in vegDict: print(vegetable, vegDict[vegetable]) #Display vegetable name and quantity