Loops: while loop in python
- Saransh Dabas
- Jun 9, 2017
- 4 min read

When we write a program, we might need to use a piece of code again and again and every time we need that piece of code we need not write it again and again instead we can use either loops or functions to use a block of code again and again. Difference between loops and functions is that we need functions when a piece of code is needed randomly in the program, I mean to say that we might not know that where that piece of code is need to be used while writing the program well in advance and we can use it whenever we feel that here we should use this function to obtain this functionality but in case of loops we know why and where we are using loops at a certain point of time. So in this tutorial we are going to see while loop in python.While loop checks a condition and then executes a set of statements specified inside the loop until the condition become false.
The syntax for while loop is:
while condition:
#set of statements to be executes
Flow control graph for while loop is:

For example if we want to print first 100 natural numbers line by line, we can do it three ways, first one is that we write 100 print statements which is obviously inconvenient for us, one other way is as we are going to use print statement again and again why not put it in a loop or we can do it using functions which we will see later.
Here is the program displaying first 100 natural numbers using while loop:
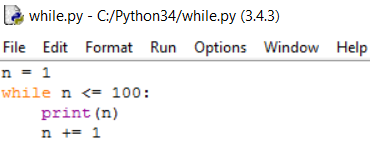
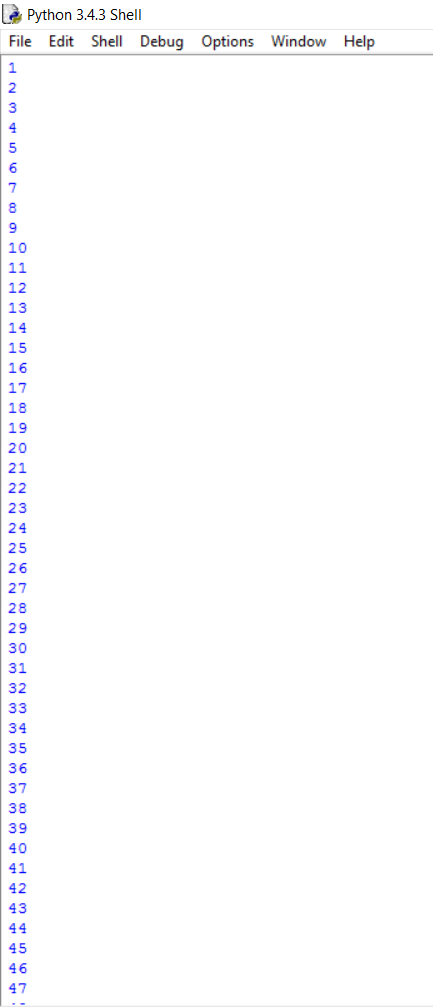
The output is larger, you can test it yourself, you can find all the codes in the code section at the end of this tutorial. You can also generalize the program by taking n value from the user and printing first n natural numbers.
In this program we first initialize the value of n as 1 then we put a while loop under the condition that n should be less than or equal to 100 and then we increment the value of n by 1, note that shorthand notation n+=1 is equivalent to n = n+1. and the code inside the loop repeats itself. Now we enter the loop the last time when n value become 100 after which n is incremented to 101 and condition become false and we come out of the loop.
One thing to note is that in the set of statement there is a statement which is responsible for eventually making the condition false(in this case it is n += 1), it is not necessary but we might end up in infinite loop if the condition doesn't become false eventually as shown:
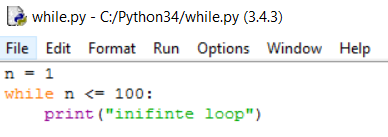
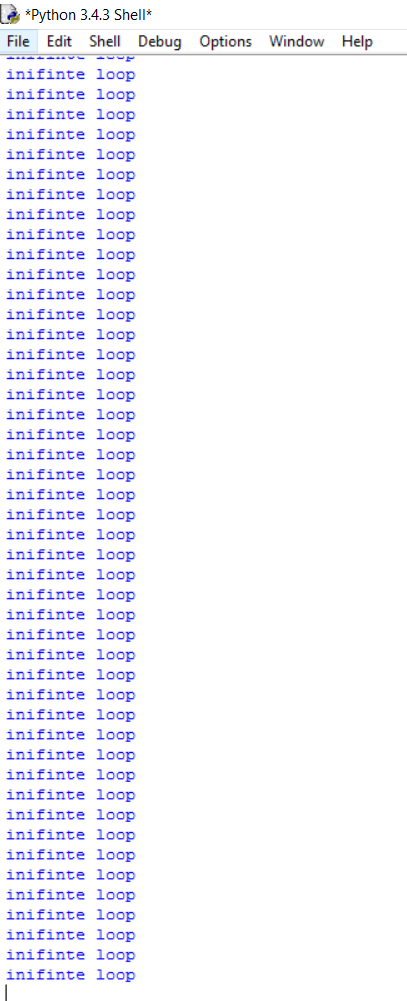
To get out of the loop you can press Ctrl + C to stop the program during execution.
There are more ways in which we can enter an infinite loop, the reason here is that n value always remain one and hence condition n<=100 always remain true, and so we stuck inside the loop forever, also if we have done something like n -= 1, the n value would keep on decrementing so we would have been in loop infinitely and there are many more ways you will learn about them when you write programs and after that you need to debug it, these infinite loops may cause you some bugs in the program. An infinite loop may be useful in network programming where the server needs to run continuously so that client process can communicate with it whenever required.
Nested loops:
We can have multiple loops inside a loop for executing a task which may require so. For example if we want to generate set of all possible (x,y) integral coordinates within some range in 1st quadrant. Here is the program for the same:
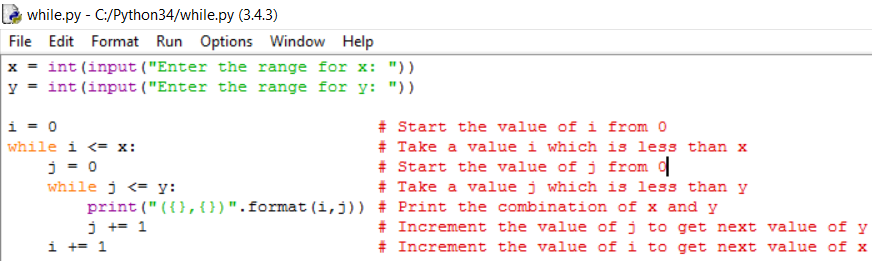
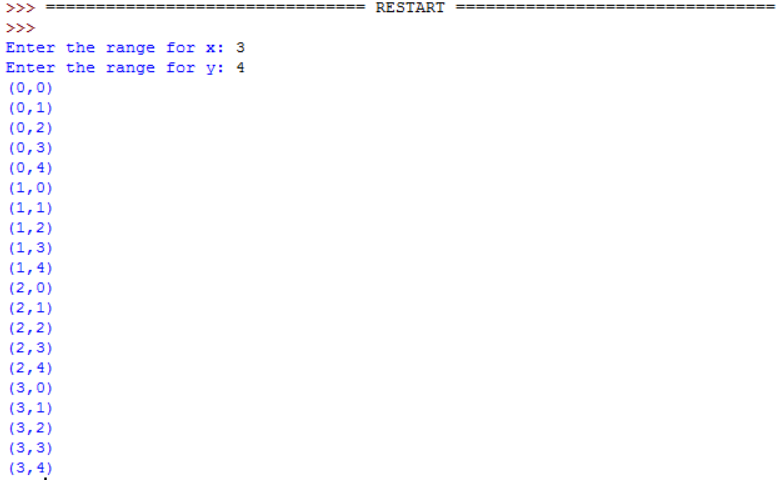
IF ELSE conditions inside while loop:
Whenever we are inside a for loop it may so happens that we need to take decision on the basis of the condition, for example if we need to check whether a number is even or odd in first say 40 whole numbers, we need two things: a loop to generate first 40 natural numbers and then an IF ELSE statement to check for the number being even or odd as shown in the following program:
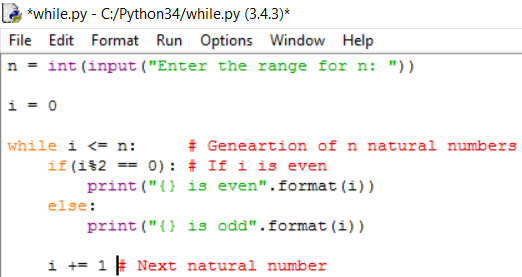
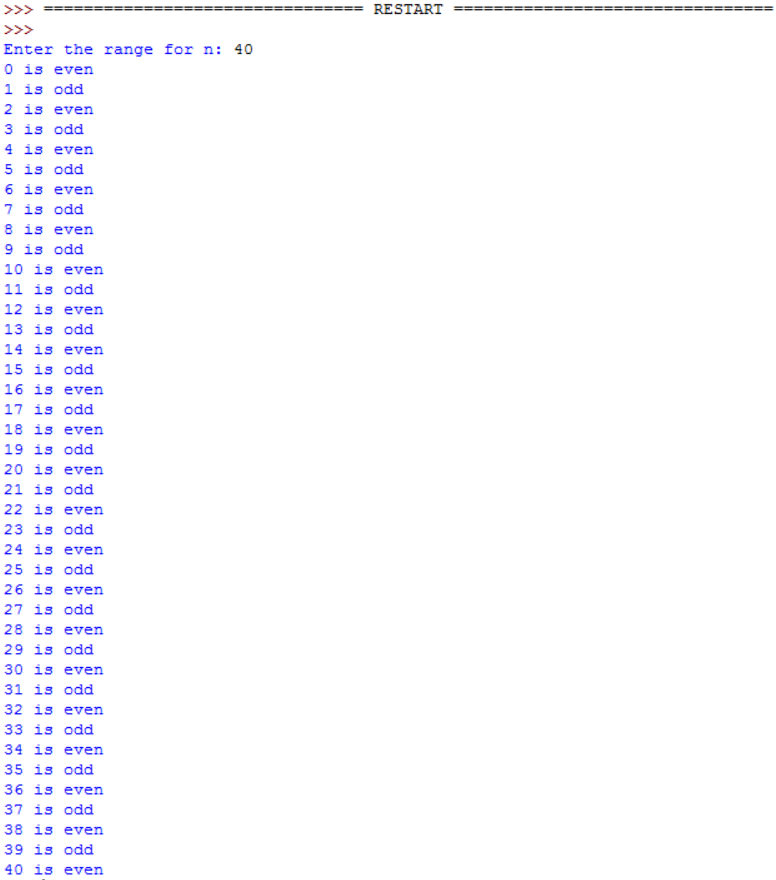
We can do much more using nested loops and if else combined like pattern generation, there is a tutorial on it in the programming problems section.
So, that's it for the tutorial and now you are able to write many programs that require loops, we will see another similar loop called as for loop in next tutorial.
Code section:
i) Printing first 100 natural numbers
n = 1 while n <= 100: print(n) n += 1
ii) Infinite loop
n = 1 while n <= 100: print("inifinte loop")
iii) Set of coordinates x = int(input("Enter the range for x: "))
y = int(input("Enter the range for y: "))
i = 0
while i <= x:
j = 0
while j <= y:
print("({},{})".format(i,j))
j += 1
i += 1
iv) Even/Odd for first n whole number:
n = int(input("Enter the range for n: "))
i = 0
while i <= n: # Geneartion of n natural numbers if(i%2 == 0): # If i is even print("{} is even".format(i)) else: print("{} is odd".format(i))
i += 1 # Next natural number