Python values, variables and datatypes

Value:
A value is a fundamental piece of data. It can be a character, word, numbers etc. Each value in python is categorized with some data type, some of the data types are strings, float, double, integer etc. If you are not sure about the data type of the value, python provides a built in function type() in which you can pass your value and it will return the data type of the value as shown in the following program:


Variables:
A variable is nothing but a name associated to a value and one of the quality of programming language to manipulate the variables is useful in writing codes to solve problems.
A variable can be assigned a value using assignment operator (=). A vaiable is nothing but an object and when we assign some value to it, python creates a link of that object with the actual value stored, when we assigned a new value the previous link is broken and a new link is created with the new value, also we can delete the reference to a number using del statement, ex del var1, var2
In python we need not explicitly say the data type of a variable before assigning it to some variable unlike C, C++ and JAVA. Just type variable = something, python will understand the data type itself and allocate memory according to the size of the data type as shown in this code:
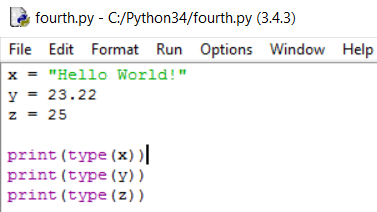

Note: Each data type has some size on the basis of which it's range is based on for example if the size of integer is 16 bits then its range varies from -2^(15) to 2^(15)-1, again the size of each data type is implementation dependent, i.e it depends on factors like OS, architecture and python version, so the size of integer on my system is maybe say 16 bit, it might be 32 bit on someone else system.
Data Types:
As you are now familiar with data types, let's see some standard data types python supports:
Numbers
Number data type store numeric value, there are three kinds of numbers python supports:
Integers (int), Floating point numbers(float) and Complex numbers(complex)
Python only supports signed integers i.e the size of integers is divided into 2 equal parts, one for positive integers(including zero) and other for negative integers and again the size is implementation dependent. ex: 23, -12, 244, -2922 etc.
Floating point numbers are the numbers with decimal point. ex-12.33
Note: You might think that 12 and 12.0 is same but in python 12 is an integer while 12.0 is a floating point number and reason why they belong to different data types is because they are implemented differently inside the system, if you want to know more about floating point numbers and how they are represented as single precision (32-bit) and double precision (64-bit) you can go here:
Complex number just like in mathematics is an ordered pair of values represented as x + yj where x and y are floating point numbers called as real numbers in mathematics and j is imaginary number.
ex: 2+3j, -1+5j, 0.3+9.5j, -2-3.2j etc.
You can perform all the operations on complex numbers as possible in mathematics
We will learn about other data types like strings, lists, sets and dictionaries in the subsequent tutorials.
Type Conversion
Sometimes we need to convert the data type of some variable, for the same we use type conversion. There are built in functions to convert one type of data to another for example int(n) converts the data type of n into an integer if possible else throws an error. The table below is a list of type conversion methods available in python:
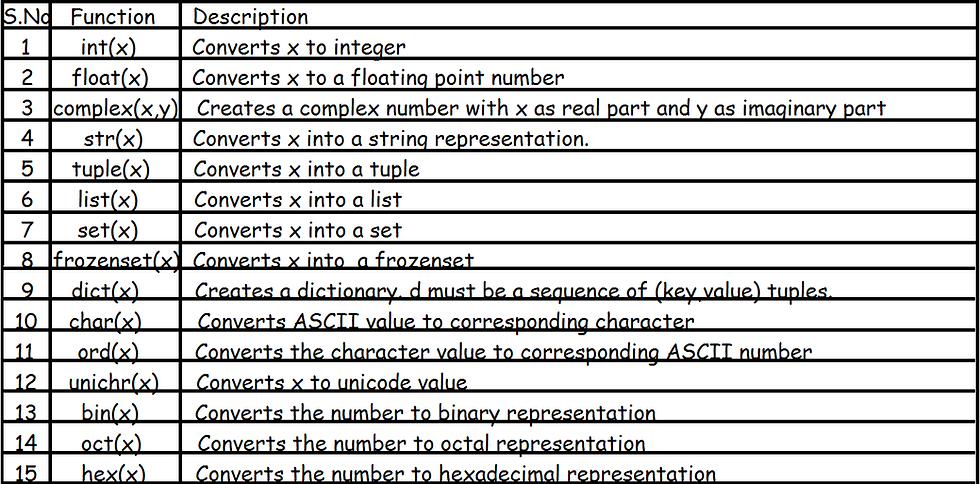
Important point:
If we use to convert from type float to int, there can be loss of information as int() will ignore fractional part completely
ex: int(4.33) -> 4
Below is a program showing the working of the above type conversions:


That's all for this one. We will learn more about data types like strings, tuples, dictionaries etc when we get into them one by one.
Code Section:
a) type() function
print(type("Hello World!")) print(type(23.22)) print(type(25))
b) Value assignment
x = "Hello World!" y = 23.22 z = 25
print(type(x)) print(type(y)) print(type(z))
c) Type Conversions
x = "65" y = "3.5"
#int(x) print("x + 3 = {}".format(int(x) + 3))
#float(y) print("y + 2.03 = {}".format(float(y) + 3))
#complex(x,y) print("Complex no = {}".format(complex(float(x), float(y))))
#str(x) print("String value = {}".format(str('234AlphaOmega')))
#set(x) print("Elements of set = {}".format(set(x)))
#frozenset(x) print("Elements of frozenset = {}".format(frozenset(x)))
#dict(x) print("Dictionary with one value {}".format({x:y}))
#chr(x) print("ASCII -> Character: {} -> {}".format(int(x), chr(int(x))))
#ord(x) print("Character -> ASCII: {} -> {}".format('-', ord('-')))
#bin(x) print("The binary representation of x is {}".format(bin(int(x))))
#oct(x) print("The octal representation of x is {}".format(oct(int(x))))
#hex(x) print("The hexadecimal representation of x is {}".format(hex(int(x))))