Operators and expressions in python
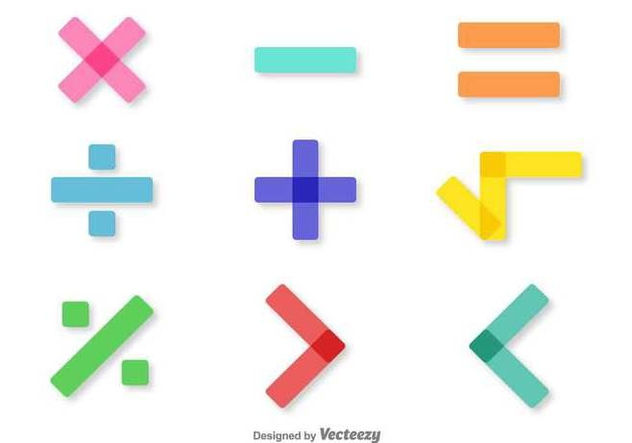
Hey guys, in this tutorial we are going to learn about various operators and expressions in python, how they are used to perform certain tasks.
All codes being described in the tutorial are given in the code section at the last
i) Basic arithmetic operators:
Arithmetic operators are used to carry out the arithmetic operations or we can say mathematical operations on single or multiple operands.
In case there are multiple operands present the order in which they are evaluated is:
Parenthesis (), Exponents (**), Multiplication (*), Division (/), Floor Division (//), Remainder (%), Addition (+), Subtraction (-)
(+) Addition (x+y)
->Used for addition of two or more operands
->This operator can also be used with strings in the sense the strings can be concatenated using + operator
->Shorthand notation: +=
(-) Subtraction (x-y)
->Subtract the right operand from the left.
->In case you want absolute value i.e positive value even when the result is negative we can use abs function.
-> Shorthand notation: -=
(*) Multiplication (x*y)
->Used for the multiplication of operands
->Shorthand notation: *=
(/) Division (x/y)
->Divides the left operand with the right one
->Shorthand notation: /=
(//) Floor Division(x//y)
->Divides the left operand by right one but returns the floor value, i.e it ignores the decimal part and returns only the integral part of the result
in case if the result is negative the floor value is calculated by subtracting 1 from the integral part
->Shorthand notation: //=
(%) Modulo (x%y)
-> Divides x with y and returns the remainder value
->Shorthand notation: %=
(**) Exponent (x**y)
->Means that x raised to the power y, or we can say it returns the value of x multiplied with itself y times
->Shorthand notation: **=
Here are two programs showing the working of operators and their shorthand notations respectively:
a)
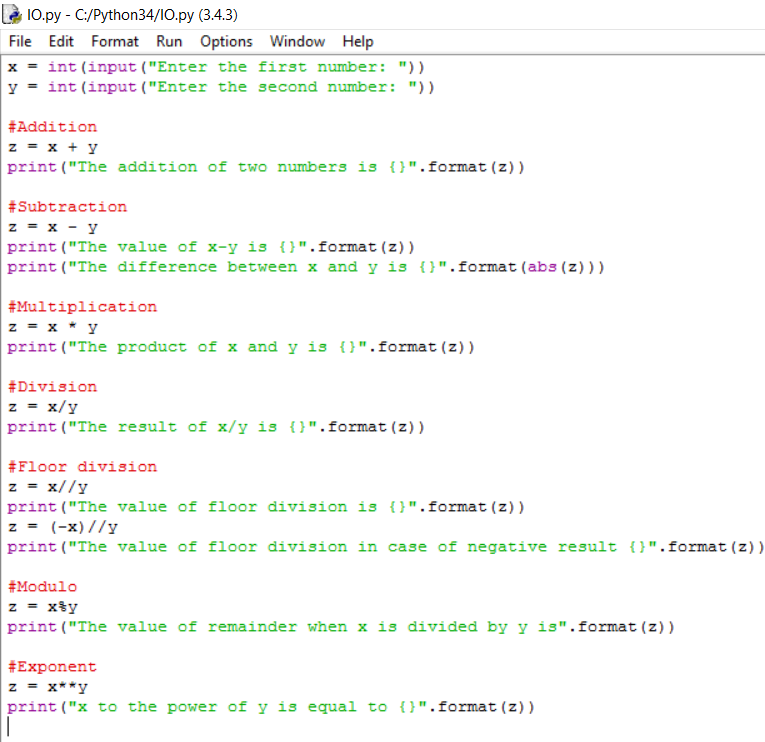

b)
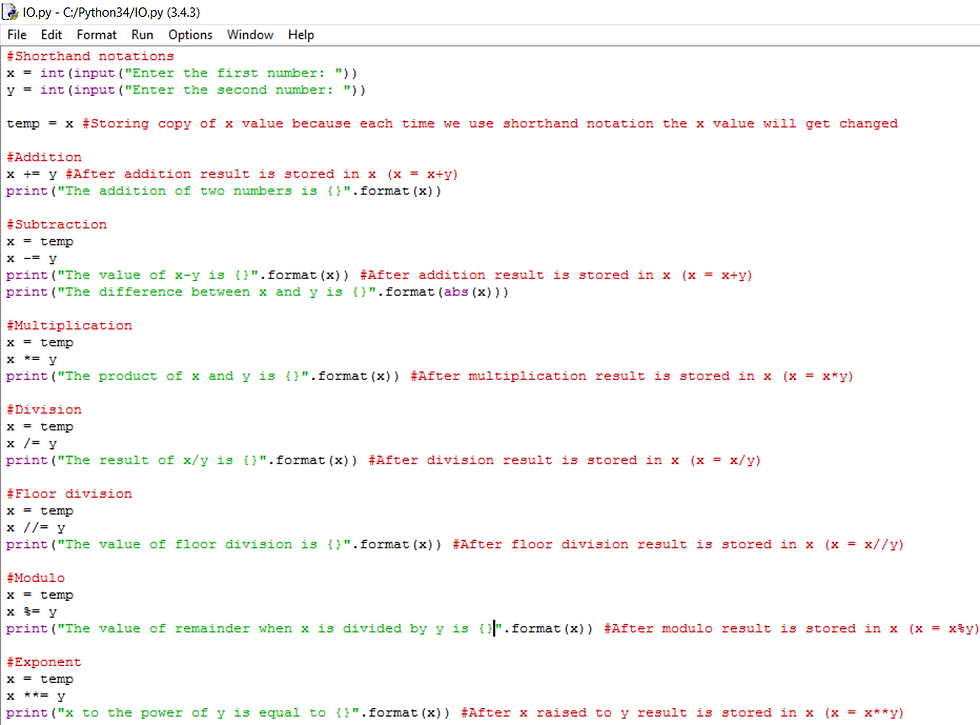
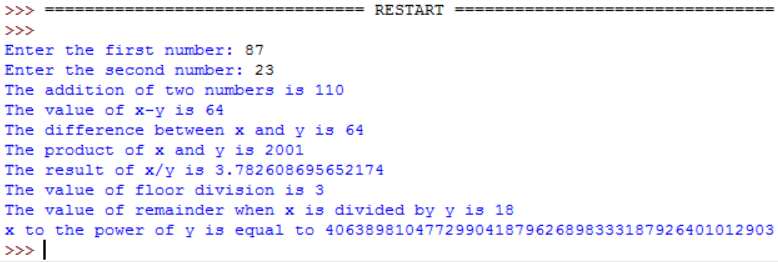
ii) Comparison operators or relation operators
These operators compares the value of operands on either sides of the operator and tells the relation between them. They return True of False value on the basis of comparison.
(==) Equal to (x == y)
->Checks whether the value of x is equal to y or not, in case it is equal it returns True else it returns False
(!=) Not equal to (x != y)
->Checks whether the value of x is not equal to x, in case it is not equal to x it returns True else it returns False
(>) Greater than (x > y)
->Checks whether the value of x is greater than y, returns True if it is greater else it returns False
->Checks if x is strictly greater than y
(>=) Greater than equal to (x >= y)
->Checks whether the value of x i greater than or equal to y, in case it is greater than or equal to y it returns True and False if x is lesser than y
(<) Less than (x < y)
->Checks whether the value of x is smaller than y, returns True if it is lesser, in case it is not the value returned is False
->Checks if x is strictly less than y
(<=) Less than or equal to (x <= y)
->Checks whether the value of x is smaller than or equal to y, in case it is smaller than o equal to y it returns True and False if x is greater than y
Here is the program showing the working of these operators:
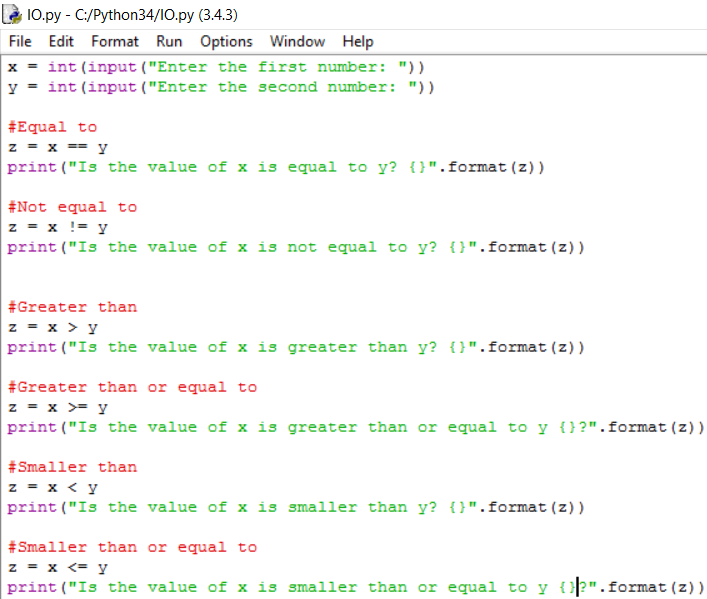
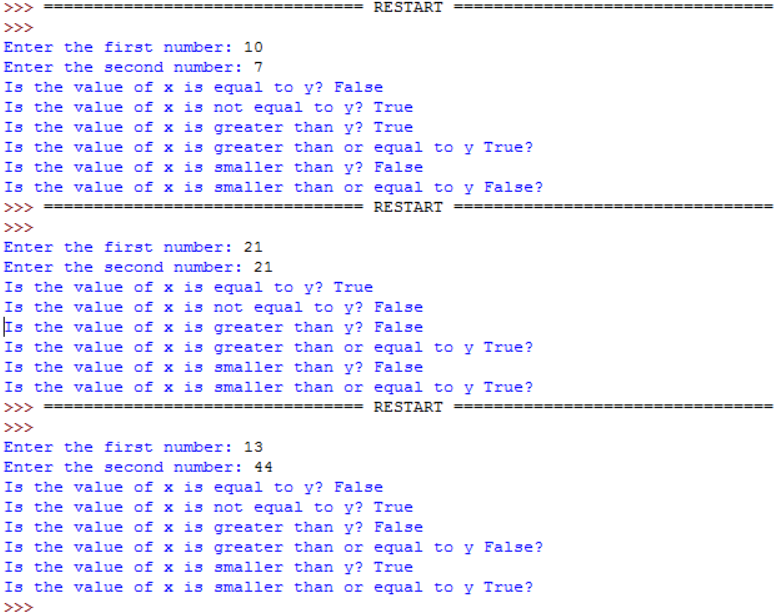
iii) Logical operators
There are three logical operators in python, these operators are used for the comparison among the boolean values.
Suppose two operands x and y are holding some boolean values i.e True or False values
a) AND (x and y)
->Returns True only when both x and y are True
Truth table for AND is:
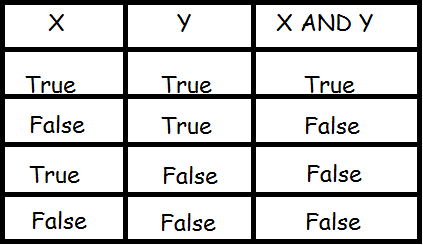
b) OR (x or y) ->Returns True when either of x or y is True
Truth table for OR is:

c) NOT (not x)
->This operator inverts the logical state of x, i.e if it is True it is converted to False and vice-versa.
Truth table for NOT is:
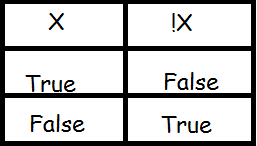
Following program shows how these operators work:

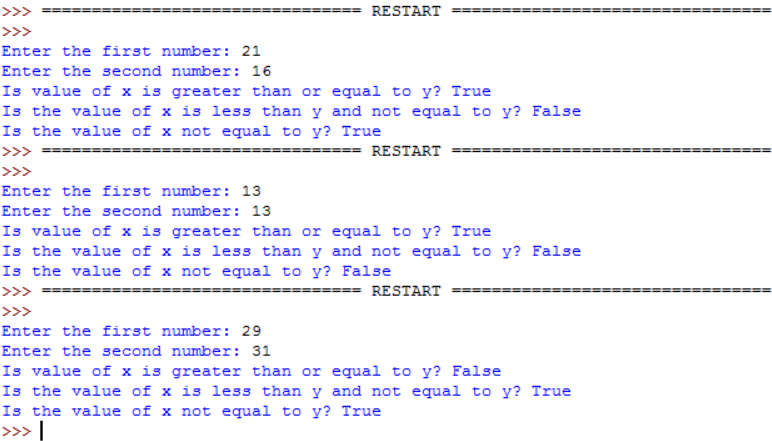
iv) Bitwise operators
Bitwise operators perform certain logical operations bit by bit on operand(s). Using bin() we can convert numbers to binary representation and see the working of these operators. There are 6 bitwise operators supported by python:
a) Binary AND (x & y)
->Takes AND of the bits of a and b bit by bit
->AND: if both the bits are 1 only then returns 1 else 0
->ex: x = 5 and y = 6
x = 0101
y = 0110
----------------
x&y = 0100
b) Binary OR (x | y)
->Takes OR of the bits of a and b, bit by bit
->OR: if any of the bits is 1 then returns 1 else 0
->ex: x = 5 and y = 6
x = 0101
y = 0110
----------------
x&y = 0111
c) Binary XOR (x ^ y)
->Takes XOR of the bits of a and b, bit by bit
->XOR: if both the bits are same then returns 0 else 1
->ex: x = 5 and y = 6
x = 0101
y = 0110
----------------
x&y = 0011
d) Binary 1's complement or NOT (~x)
->Take the complement of each bit
->NOT: if the bit is 0 then returns 1 else 0
->ex: x = 5 and y = 6
x = 0101
----------------
~x = 1010
e) Binary left shift (x << k)
->Shifts the binary number by k places to the left
->Append k zeros to the end of the number
x = 0101
----------------
x<<2 = 010100
e) Binary right shift (x >> k)
->Shifts the binary number by k places to the right
->Deletes k bits from the back
x = 0101
----------------
x>>2 = 0001
The following program shows the working of bitwise operators:


When we encounter more than one operator in a single expression then there must be some precedence rules which must be followed in order to get correct results.
ex 2*4+1 = 9 and not 10, similarly 3+2/2 = 4 not 2.5 etc, so here is the table for precedence of operators in python:
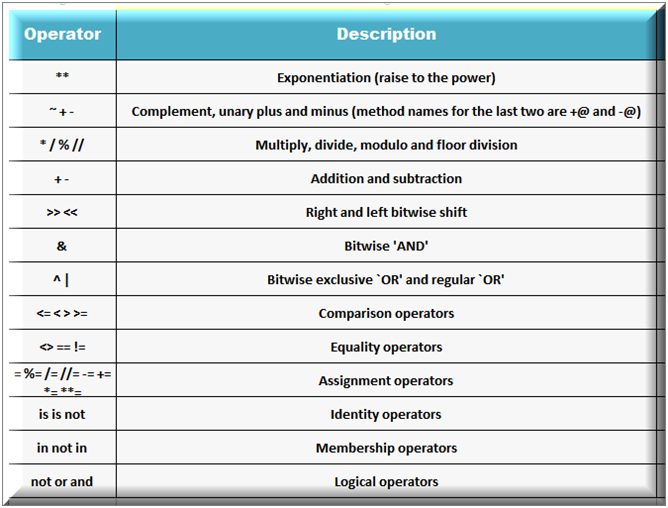
There is one more thing called associativity, when we have two or more operators of same precedence then python follow left associativity rule i.e operators are evaluated from left to right
ex: 22*100//60 = (22*100)//60 = 36 --->Left associativity (Correct answer)
22*100//60 = 22*(100//60) = 22 --->Right associativity (Wrong answer)
These were the basic operators in python, there are more operators and we will see them in upcoming next tutorials when we are learning about strings and lists.
Code section:
a) Arithmetic operators:
x = int(input("Enter the first number: ")) y = int(input("Enter the second number: "))
#Addition z = x + y print("The addition of two numbers is {}".format(z))
#Subtraction z = x - y print("The value of x-y is {}".format(z)) print("The difference between x and y is {}".format(z))
#Multiplication z = x * y print("The product of x and y is {}".format(z))
#Division z = x/y print("The result of x/y is {}".format(z))
#Floor division z = x//y print("The value of floor division is {}".format(z)) z = (-x)//y print("The value of floor division in case of negative result {}".format(z))
#Modulo z = x%y print("The value of remainder when x is divided by y is".format(z))
#Exponent z = x**y print("x is raised to y the value is {}".format(z))
#Shorthand notations x = int(input("Enter the first number: ")) y = int(input("Enter the second number: "))
temp = x #Storing copy of x value because each time we use shorthand notation the x value will get changed
#Addition x += y #After addition result is stored in x (x = x+y) print("The addition of two numbers is {}".format(x))
#Subtraction x = temp x -= y print("The value of x-y is {}".format(x)) #After addition result is stored in x (x = x+y) print("The difference between x and y is {}".format(abs(x)))
#Multiplication x = temp x *= y print("The product of x and y is {}".format(x)) #After multiplication result is stored in x (x = x*y)
#Division x = temp x /= y print("The result of x/y is {}".format(x)) #After division result is stored in x (x = x/y)
#Floor division x = temp x //= y print("The value of floor division is {}".format(x)) #After floor division result is stored in x (x = x//y)
#Modulo x = temp x %= y print("The value of remainder when x is divided by y is {}".format(x)) #After modulo result is stored in x (x = x%y)
#Exponent x = temp x **= y print("x to the power of y is equal to {}".format(x)) #After x raised to y result is stored in x (x = x**y)
b) Comparison operators
x = int(input("Enter the first number: ")) y = int(input("Enter the second number: "))
#Equal to z = x == y print("Is the value of x is equal to y? {}".format(z))
#Not equal to z = x != y print("Is the value of x is not equal to y? {}".format(z))
#Greater than z = x > y print("Is the value of x is greater than y? {}".format(z))
#Greater than or equal to z = x >= y print("Is the value of x is greater than or equal to y {}?".format(z))
#Smaller than z = x < y print("Is the value of x is smaller than y? {}".format(z))
#Smaller than or equal to z = x <= y print("Is the value of x is smaller than or equal to y {}?".format(z))
c) Logical operators
x = int(input("Enter the first number: ")) y = int(input("Enter the second number: "))
#Some boolean values p = x == y q = x != y r = x > y t = x < y
#Comparison of boolean values
print("Is value of x is greater than or equal to y? {}".format(p or r))
print("Is the value of x is less than y and not equal to y? {}".format(q and t))
print("Is the value of x not equal to y? {}".format(not p))
d) Bitwise operators
x = int(input("Enter the value for x: ")) y = int(input("Enter the value for y: "))
print("The binary representation of x is",bin(x)) print("The binary representation of x is",bin(y))
#AND print("Bitwise x AND y = {}, in binary: {}".format(x & y, bin(x & y)))
#OR print("Bitwise x OR y = {}, in binary: {}".format(x | y, bin(x | y)))
#XOR print("Bitwise x XOR y = {}, in binary: {}".format(x ^ y, bin(x ^ y)))
#1's complement print("1's complement of x = {}, in binary: {}".format(~x, bin(~x)))
#Left shift print("Left shifting x = {}, in binary: {}".format(x<<2, bin(x<<2)))
#Right shif print("Right shifting y = {}, in binary: {}".format(x>>2, bin(x>>2)))