Input/Output in python
- Saransh Dabas
- May 31, 2017
- 2 min read

Input:
Taking input in python is very simple, we use built-in input() function to take input from the user. We can either specify the type of input we are taking example int(input()) or by default python takes everything as string, later on we can convert the type into the way we want. Also we can type in " " as parameters for input functions to display something on screen asking user to get some value, we can also concatenate strings using + operator and display using print statement
The above points will be cleared from the below example:
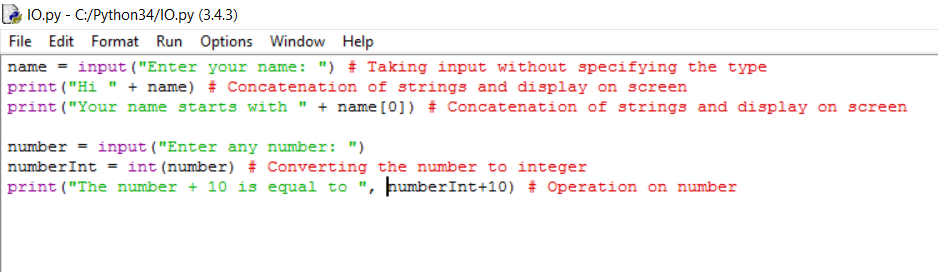

We can also take input by specifying the type:
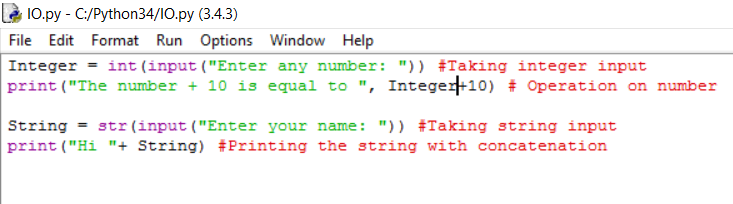

If we try to give input which doesn't match the type, python will throw an error, note that string can accept even numbers because string can be anything.
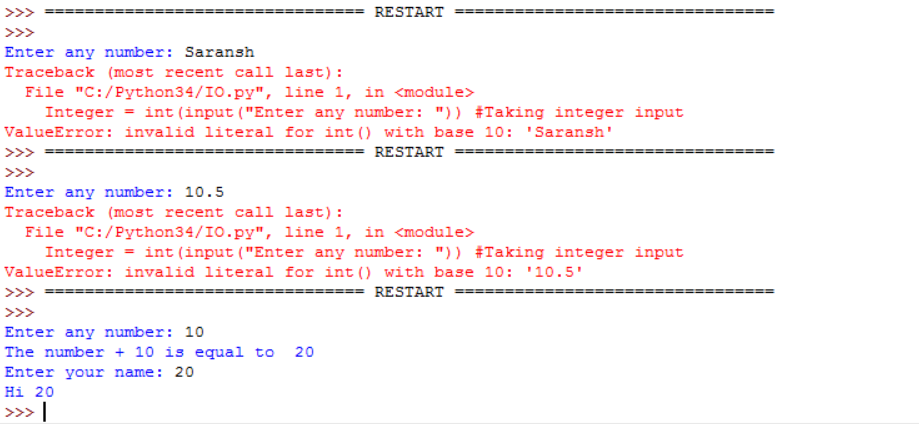
Taking multiple inputs in a single line:
Here is a small program which calculates the distance of a point from the origin after taking x, y, z coordinates as input but be careful, don't mention type as int x, y, z = int(input()) as it will throw an error.
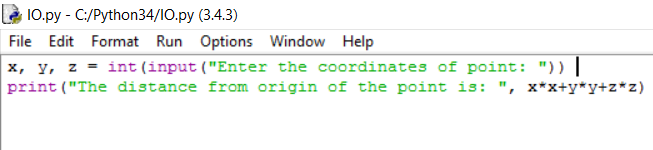
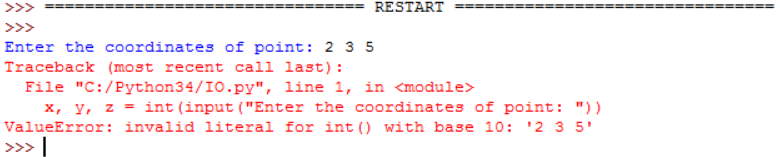
The reason for this error is that as there are three inputs in a line 2 3 5, the compiler gets confused that what is 2 3 5? a single number or how should I split this number.
So we need to take input as it is and then convert them according to our requirements. The split function is used to split the values after one blank space
This point will be clear from the following example:


Output:
The built-in function print() can be used to display something on the screen, inside print statement you can perform arithematic operations on numbers, concatenation on strings, indexing and slicing on strings which we will see in string section.
Simple print statement:

Arithematic and string operations in print statement:


Note that we need to convert Age from integer to string so that in can qualify for string concatenation (+) operator.
We can do the same thing in an easier way using .format() function which is defined in built in libraries for strings.
values are substituted in order in place of {}, this functionality is analogous to printf("The value of c is %d", c) of C language in which c value is substituted in place of %d.
It will be clear from the following example:
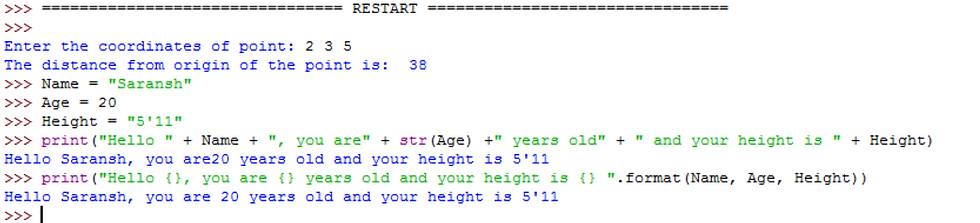
Note that we need not change the type of Age to string as .format function takes care of that for us.
So, that was the introduction on the input() and print() functions of python, we will get to learn more about them as we write more programs in the upcoming tutorials.
Comments