NUMBERS from numbers.
- Saransh Dabas
- Feb 5, 2017
- 6 min read
The task is very simple
* Input :
82737
* Output :

Isn't that amazing, here is how to do it
Printing the numbers one after another vertically is very easy task but that's what not we are looking for, we need numbers to be printed horizontally one after the other and so one way to the same is to define a function for every line for each number.
For example 8 :
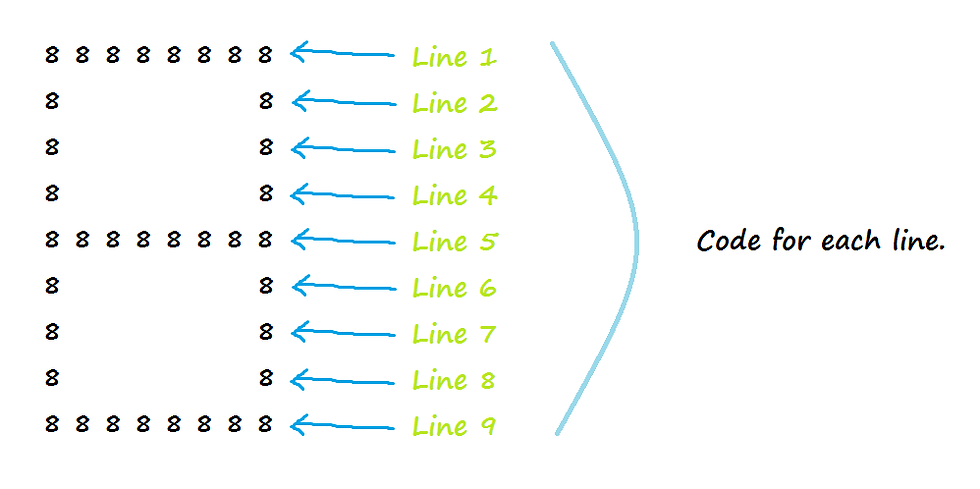
But that would be tedious as we have to define 9 functions for each number total 90 functions and if a function takes 10 lines then there would be 900 lines of code for only those functions, but as a good programmer we must try to reduce the number of lines of code.
One thing to observe is that some of the lines are same and so we need not write code redundantly, as shown below :
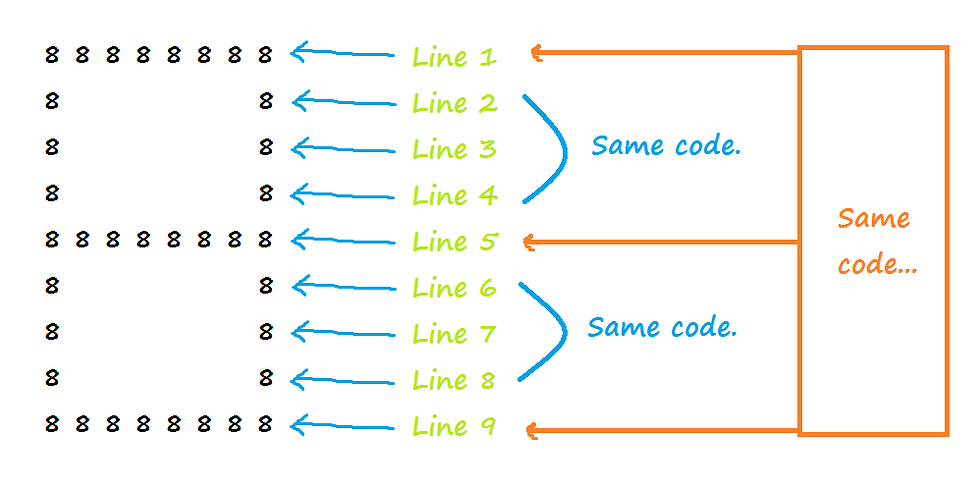
So, the is problem is less logical and more copy/paste type, there will be a total of 24 functions if we go in this way.
I will be explaining all the different functions, rest of them will be copy paste :
a) Number : 0 and Line : 1 and 9
Just print "0" 5 times in a row, I am using 5, you can use any number you want.
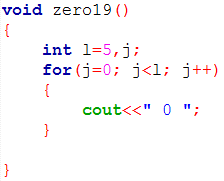
b) Number : 0 and Line : 2,3,4,5,6,7,8
Print for the first and last place else print white space.
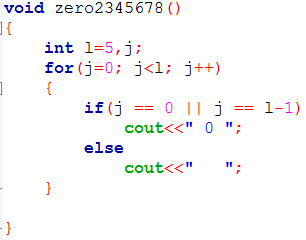
c) Number : 1
Print only one character for each line.
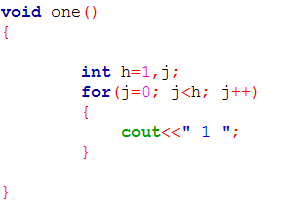
d) Number : 2 and Line : 1,5,9
Same as number 0 and line 1 & 9;
e) Number : 2 and Line : 2,3,4
Print only the last element.

f) Number : 2 and Line : 6,7,8
Print only the first element
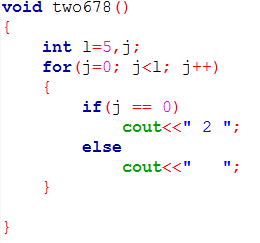
g) Number : 3 and Line : 1,5,9
Same as Number 0 and Line 1 & 9.
h) Number : 3 and Line : 234678
Same as Number : 2 and Line : 2,3,4
i) Number : 4 and Line : 1,2,3,4
Same as Number : 0 and Line : 2,3,4,5,6,7,8
j) Number : 4 and Line : 5
Same as Number : 0 and Line : 1 and 9
k) Number : 4 and Line : 6,7,8,9
Same as Number : 2 and Line : 2,3,4
l) Number : 5 and Line : 1,5,9
Same as Number : 0 and Line : 1,9
m) Number : 5 and Line : 2,3,4
Same as Number : 2 and Line : 6,7,8
n) Number : 5 and Line : 6,7,8
Same as Number : 2 and Line : 2,3,4
o) Number : 6 and Line : 1,5,9
Same as Number :.0 and Line : 1,9
p) Number : 6 and Line :2,3,4
Same as Number : 2 and Line : 6,7,8
q) Number : 6 and Line : 6,7,8
Same as Number : 0 and Line : 2,3,4,5,6,7,8
r) Number : 7 and Line : 1
Same as Number 0 and Line 1 & 9
s) Number : 8 and Line : 1,5,9
Same as Number 0 and Line 1 & 9
t) Number : 8 and Line : 2,3,4,6,7,8
Same as Number : 0 and Line : 2,3,4,5,6,7,8
u) Number : 9 and Line : 1,5,9
Same as Number 0 and Line 1 & 9.
v) Number : 9 and Line : 2,3,4
Same as Number : 0 and Line : 2,3,4,5,6,7,8
w) Number : 9 and Line : 6,7,8
Same as Number : 2 and Line : 2,3,4
After having these functions we need to do 2 more things :
1) Extracting each digit from the number
We take modulo 10 of the number n and for the next iteration n becomes n/2, we do this because after diving by 10 the remainder is the last digit of that number (talking about decimal system) and after getting the last digit we no longer required that so we divide by 10 which eliminates the last digit
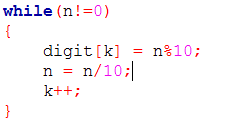
2) Calling the required function
The digits must be printed line by line in order to tuck them in horizontal fashion, to do the same we use 2 for loops, the outer for loop will take care of i starting from 1st line to 9th line. The inner one will take care of which digit needs to be printed, hence together they will print every line for every number one by one.
As we get the sequence of numbers in reverse order (i.e from last digits to the first one) we are taking j value in reverse manner in order to get the correct sequence of digits.
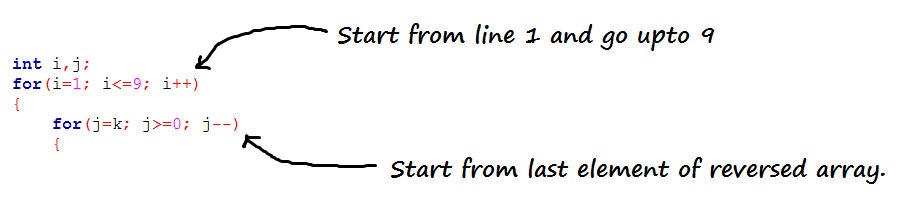
Then on the basis of the value of i and j the number and the line is decided and the function is called accordingly
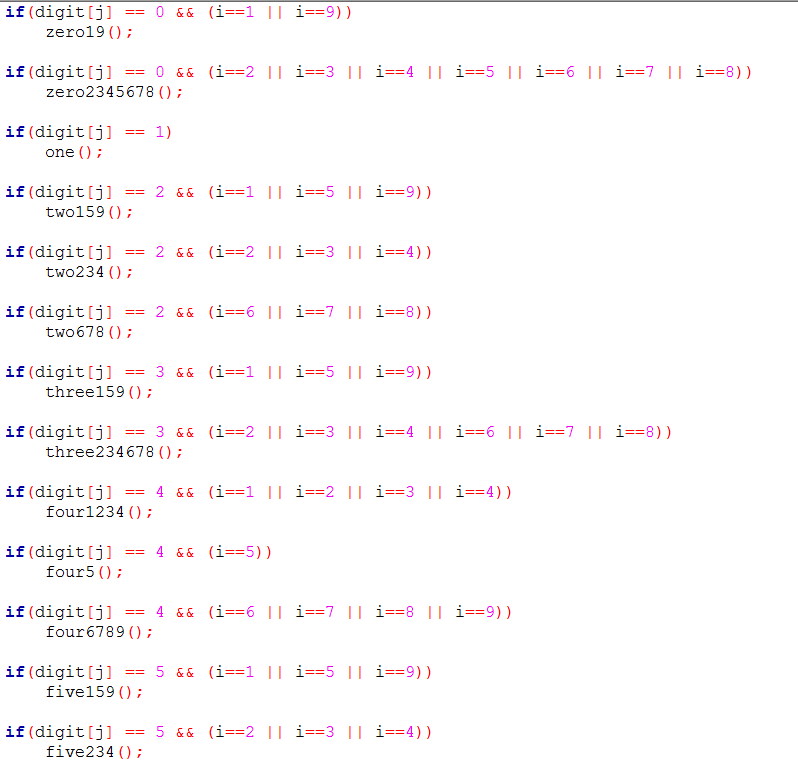
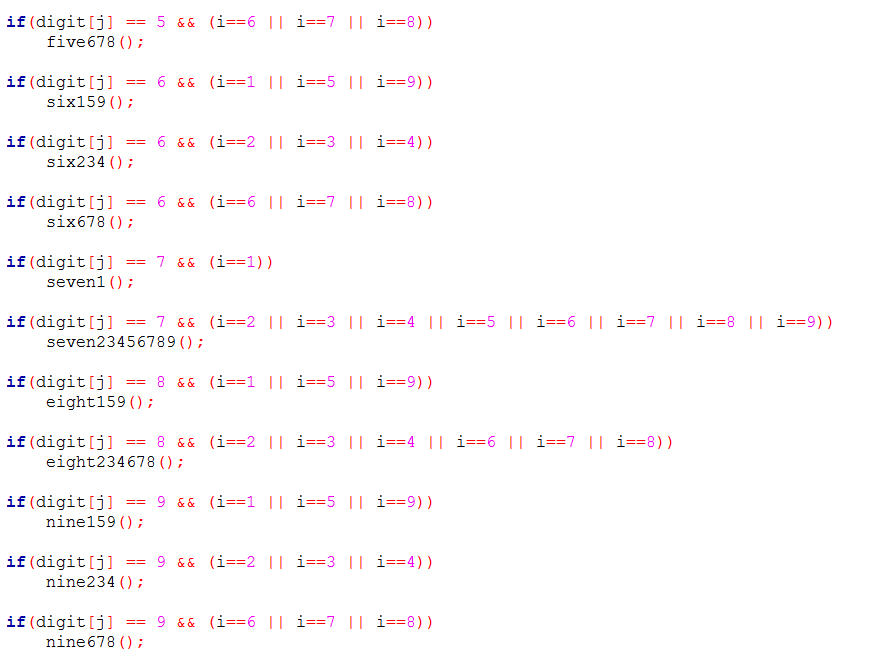
There you go, we have all the things we need here comes some samples and full code:
Full C++ implementation/ Code :
using namespace std;
void zero19() { int l=5,j; for(j=0; j<l; j++) { cout<<" 0 "; }
}
void zero2345678() { int l=5,j; for(j=0; j<l; j++) { if(j == 0 || j == l-1) cout<<" 0 "; else cout<<" "; }
}
void one() {
int h=1,j; for(j=0; j<h; j++) { cout<<" 1 "; }
}
void two159() {
int l=5,j; for(j=0; j<l; j++) { cout<<" 2 "; } }
void two234() { int l=5,j; for(j=0; j<l; j++) { if(j == l-1) cout<<" 2 "; else cout<<" "; }
}
void two678() { int l=5,j; for(j=0; j<l; j++) { if(j == 0) cout<<" 2 "; else cout<<" "; }
}
void three159() { int l=5,j; for(j=0; j<l; j++) { cout<<" 3 "; }
}
void three234678() { int l=5,j; for(j=0; j<l; j++) { if(j == l-1) cout<<" 3 "; else cout<<" "; }
}
void four1234() { int l=5,j; for(j=0; j<l; j++) { if(j == 0 || j == l-1) cout<<" 4 "; else cout<<" "; }
}
void four5() { int l=5,j; for(j=0; j<l; j++) { cout<<" 4 "; }
}
void four6789() { int l=5,j; for(j=0; j<l; j++) { if(j == l-1) cout<<" 4 "; else cout<<" "; }
}
void five159() { int l=5,j; for(j=0; j<l; j++) { cout<<" 5 "; }
}
void five234() { int l=5,j; for(j=0; j<l; j++) { if(j == 0) cout<<" 5 "; else cout<<" "; }
}
void five678() { int l=5,j; for(j=0; j<l; j++) { if(j == l-1) cout<<" 5 "; else cout<<" "; }
}
void six159() { int l=5,j; for(j=0; j<l; j++) { cout<<" 6 "; }
}
void six234() { int l=5,j; for(j=0; j<l; j++) { if(j == 0) cout<<" 6 "; else cout<<" "; } }
void six678() { int l=5,j; for(j=0; j<l; j++) { if(j == 0 || j == l-1) cout<<" 6 "; else cout<<" "; }
}
void seven1() { int l=5,j; for(j=0; j<l; j++) { cout<<" 7 "; }
}
void seven23456789() { int l=5,j; for(j=0; j<l; j++) { if(j == l-1) cout<<" 7 "; else cout<<" "; }
}
void eight159() { int l=5,j; for(j=0; j<l; j++) { cout<<" 8 "; }
}
void eight234678() { int l=5,j; for(j=0; j<l; j++) { if(j == 0 || j == l-1) cout<<" 8 "; else cout<<" "; } }
void nine159() { int l=5,j; for(j=0; j<l; j++) { cout<<" 9 "; }
}
void nine234() { int l=5,j; for(j=0; j<l; j++) { if(j == 0 || j == l-1) cout<<" 9 "; else cout<<" "; }
}
void nine678() { int l=5,j; for(j=0; j<l; j++) { if(j == l-1) cout<<" 9 "; else cout<<" "; }
}
int main() {
int n; cout<<"Enter the number "; cin>>n; cout<<endl<<endl;
int temp = n; int counter=0,firstDigit,k=0; while(temp!=0) { counter++; temp = temp/10; }
int digit[counter]; int p,q; while(n!=0) { digit[k] = n%10; n = n/10; k++; }
int i,j; for(i=1; i<=9; i++) { for(j=k; j>=0; j--) {
if(digit[j] == 0 && (i==1 || i==9)) zero19();
if(digit[j] == 0 && (i==2 || i==3 || i==4 || i==5 || i==6 || i==7 || i==8)) zero2345678();
if(digit[j] == 1) one();
if(digit[j] == 2 && (i==1 || i==5 || i==9)) two159();
if(digit[j] == 2 && (i==2 || i==3 || i==4)) two234();
if(digit[j] == 2 && (i==6 || i==7 || i==8)) two678();
if(digit[j] == 3 && (i==1 || i==5 || i==9)) three159();
if(digit[j] == 3 && (i==2 || i==3 || i==4 || i==6 || i==7 || i==8)) three234678();
if(digit[j] == 4 && (i==1 || i==2 || i==3 || i==4)) four1234();
if(digit[j] == 4 && (i==5)) four5();
if(digit[j] == 4 && (i==6 || i==7 || i==8 || i==9)) four6789();
if(digit[j] == 5 && (i==1 || i==5 || i==9)) five159();
if(digit[j] == 5 && (i==2 || i==3 || i==4)) five234();
if(digit[j] == 5 && (i==6 || i==7 || i==8)) five678();
if(digit[j] == 6 && (i==1 || i==5 || i==9)) six159();
if(digit[j] == 6 && (i==2 || i==3 || i==4)) six234();
if(digit[j] == 6 && (i==6 || i==7 || i==8)) six678();
if(digit[j] == 7 && (i==1)) seven1();
if(digit[j] == 7 && (i==2 || i==3 || i==4 || i==5 || i==6 || i==7 || i==8 || i==9)) seven23456789();
if(digit[j] == 8 && (i==1 || i==5 || i==9)) eight159();
if(digit[j] == 8 && (i==2 || i==3 || i==4 || i==6 || i==7 || i==8)) eight234678();
if(digit[j] == 9 && (i==1 || i==5 || i==9)) nine159();
if(digit[j] == 9 && (i==2 || i==3 || i==4)) nine234();
if(digit[j] == 9 && (i==6 || i==7 || i==8)) nine678();
cout<<" "; } cout<<endl; }
cout<<endl<<endl;
}
NOTE : Careful while entering the number! Take care of integer bounds and if you want large number to be printed change the datatype to long, if more than that use long long.
Sample INPUT/OUTPUT :
283761 :
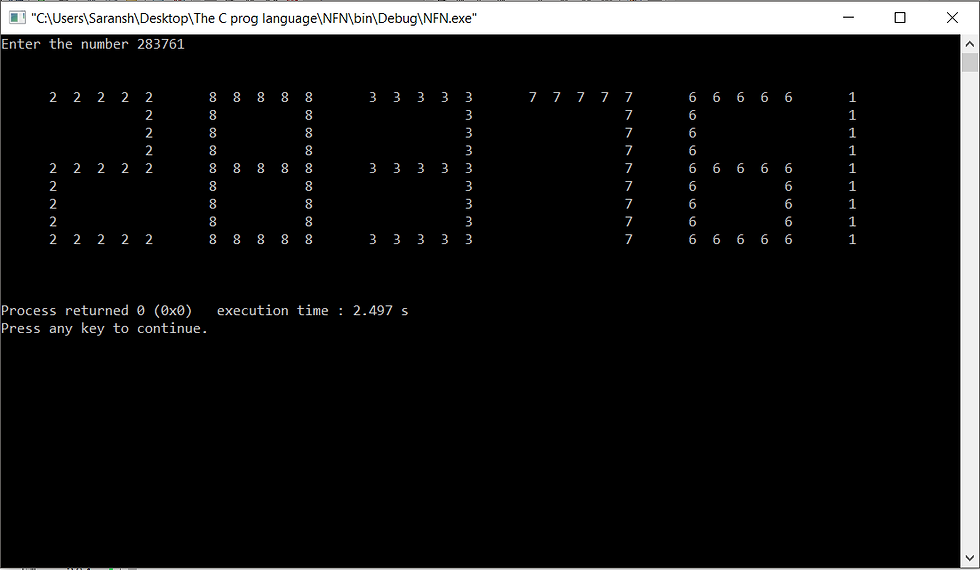
98575122 :
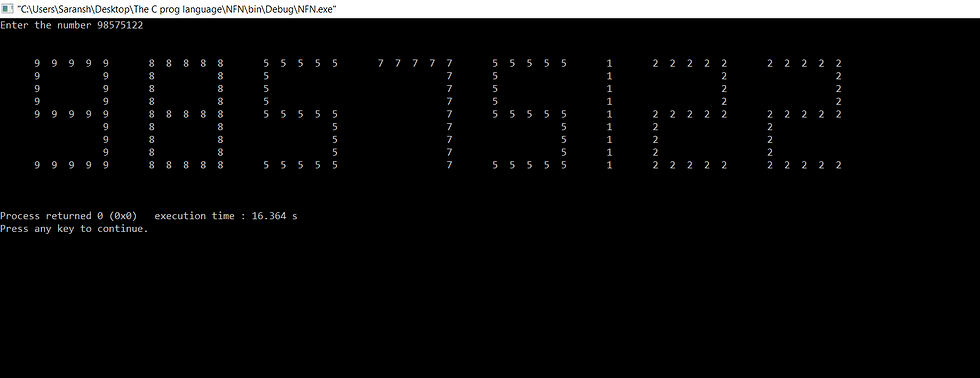
10928022 :

454132 :

Comentários