Learn Android App dev: Using Notifications
Hey guys! In this post, I'll be guiding on how to show Notifications for your Android App. Well, these days every other app wants to show Notifications to make the user make use of the App frequently. Everyone of us know that the notifications will be visible in the notification panel of your Android phone. These notifications may comprise of title, small icon and description of the notification sometimes.
So, here are the steps on how to use and show Notifications:
1. First of all, drag a button in your layout which will be used to show the Notifications in the notification panel.
2. Now declare the button in the MainActivity class.
3. Now in the OnCreate method. create a new OnClickListener for the button. In it, create a new Intent which will be used for showing the Notification.
4. We also need to create one PendingIntent object which will be used to perform some action when we click on the Notification.
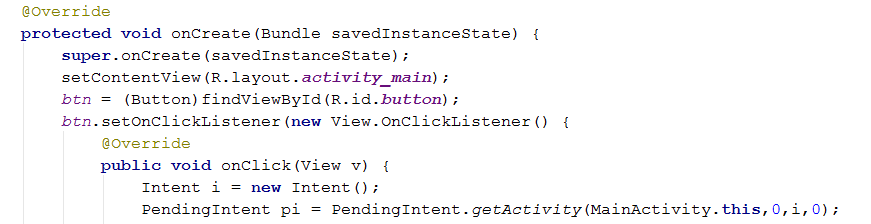
5. Now, we have to design how our Notification would look like. So for that, we need a new Notification builder. We will use pre-defined functions which Android Studio provides us for designing the Notification.
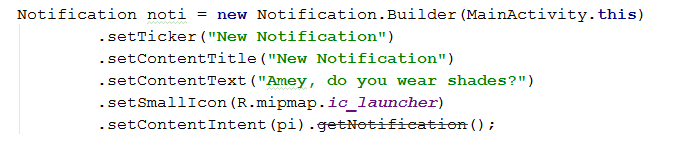
6. Now we will use some flags for making the Phone vibrate when the notification pops up and will get automatically cancelled when we click it.

7. Now we need to use the Notification manager to show the Notification. What notification manager does is it creates a notification in your Notification bar.
Use the following syntax to get the notification.

Java Code:
package com.example.ameylokhande.usingnotification;
import android.app.Notification; import android.app.NotificationManager; import android.app.PendingIntent; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button;
public class MainActivity extends AppCompatActivity { private static Button btn;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btn = (Button)findViewById(R.id.button); btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent i = new Intent(); PendingIntent pi = PendingIntent.getActivity(MainActivity.this,0,i,0); Notification noti = new Notification.Builder(MainActivity.this) .setTicker("New Notification") .setContentTitle("New Notification") .setContentText("Amey, do you wear shades ") .setSmallIcon(R.mipmap.ic_launcher) .setContentIntent(pi).getNotification();
noti.flags = Notification.DEFAULT_VIBRATE; noti.flags = Notification.PRIORITY_HIGH; noti.flags = Notification.FLAG_AUTO_CANCEL; NotificationManager notimanag = (NotificationManager)getSystemService(NOTIFICATION_SERVICE); notimanag.notify(0,noti); } }); } }