Learn Android App Dev: Using SeekBar
- Amey Lokhande
- Feb 2, 2017
- 2 min read
Hey there! This is Amey Lokhande. In this post, I'll be writing about how to use a SeekBar in your App and we will make an App which will show the progress of a SeekBar by changing it constantly. Well, SeekBars are generally used when someone wants to know about the what percent a quantity is of other or simply to know the progress of any activity.
For ex, you can use SeekBars in an App which will show the Attendance of the students in a college. Thats it!
Here are the steps on how to use a SeekBar:
1. First of all, design the layout of your App. All you need to do is drag a SeekBar widget and some TextViews to show the amount of SeekBar covered. So your app will somewhat look like:
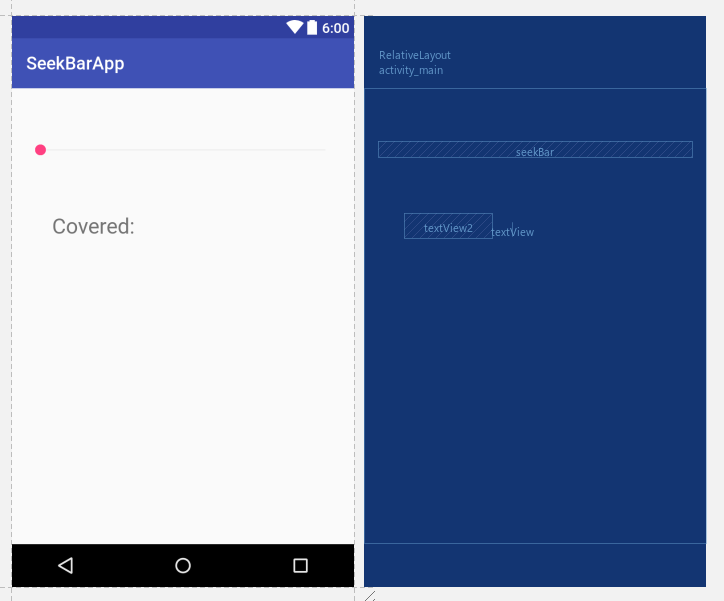
2. Now lets design the Java code. First of all, declare the SeekBar and those two TextViews in your MainActivity class.

3. Now lets create a function for showing the SeekBar progress in the textView on changing the SeekBar thumb. In that method cast your SeekBar and TextView variables. Now set the text inside the TextView using some in-built functions.

4. Now lets create a method for SetSeekBarChangeListener. Create a new OnSeeBarChangeListener argument in it. Then Android Studio will automatically generate some code for you. This is will be the Code which will be generated by Android Studio automatically.
myseekbar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { }
@Override public void onStartTrackingTouch(SeekBar seekBar) { }
@Override public void onStopTrackingTouch(SeekBar seekBar) { }
5. We have to add some more code to these methods for showing the progress of the Seekbar and showing some Toast messages. For displaying the Progress we will use some in-built functions. The syntax is given below.
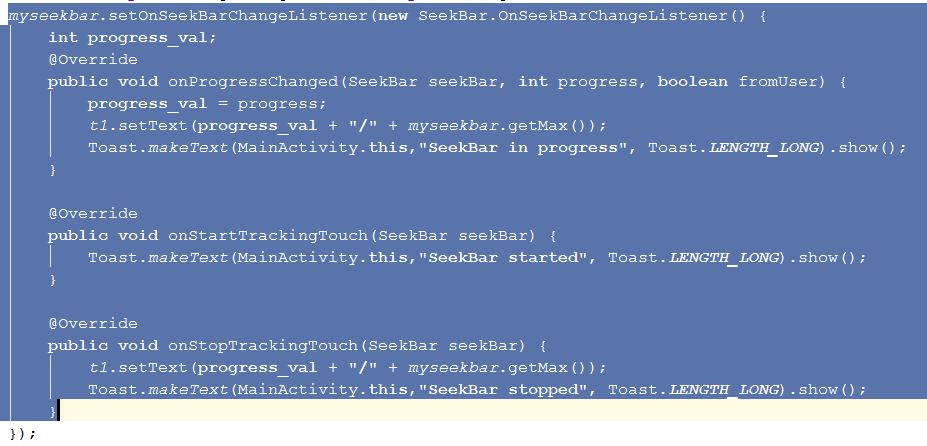
Here I have declared a variable called as progress_val for which will store the Progress of the SeekBar and rest of the code is self-explainable.
6. Now all you need is to call your method inside the OnCreate method. Thats all! Now you can run your App.
Here's a screenshot of the running app:
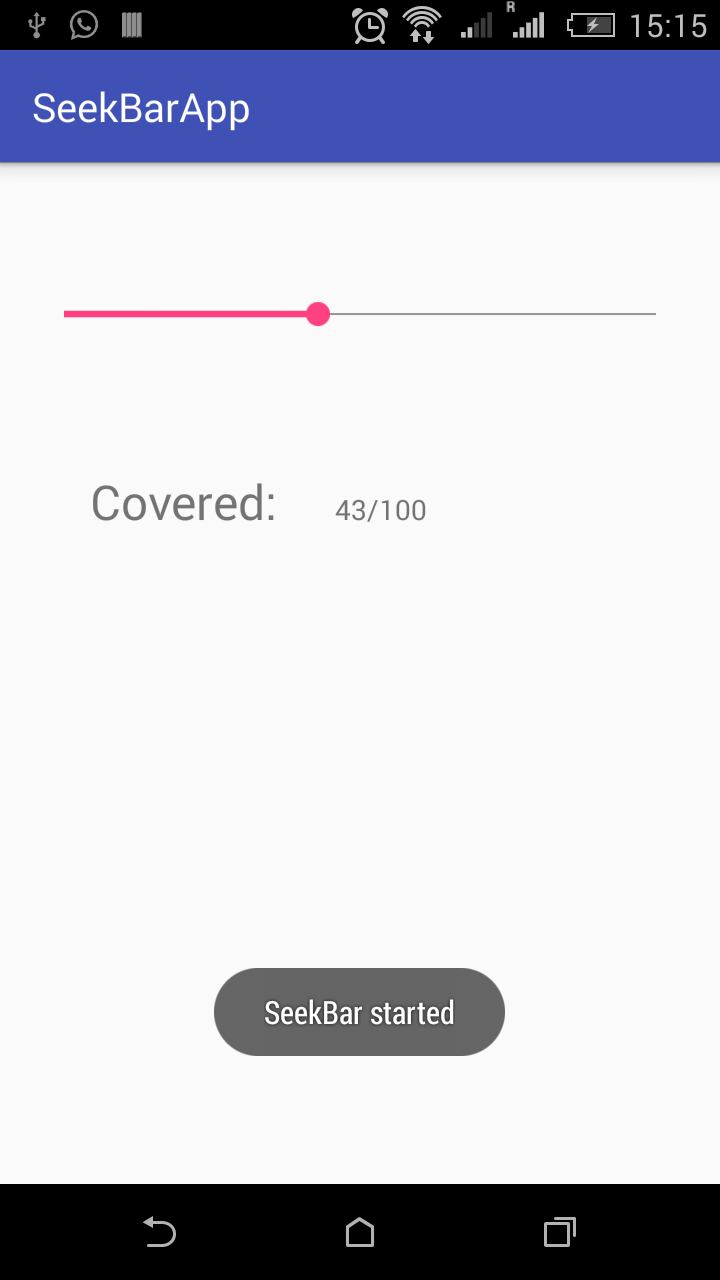
Java Code:
package com.example.ameylokhande.seekbarapp;
import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.SeekBar; import android.widget.TextView; import android.widget.Toast;
public class MainActivity extends AppCompatActivity { private static SeekBar myseekbar; private static TextView t1;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Seekbarchange(); } public void Seekbarchange(){ myseekbar = (SeekBar)findViewById(R.id.seekBar); t1 = (TextView)findViewById(R.id.textView);
t1.setText(myseekbar.getProgress()+"/"+myseekbar.getMax()); myseekbar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { int progress_val; @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { progress_val = progress; t1.setText(progress_val + "/" + myseekbar.getMax()); Toast.makeText(MainActivity.this,"SeekBar in progress", Toast.LENGTH_LONG).show(); }
@Override public void onStartTrackingTouch(SeekBar seekBar) { Toast.makeText(MainActivity.this,"SeekBar started", Toast.LENGTH_LONG).show(); }
@Override public void onStopTrackingTouch(SeekBar seekBar) { t1.setText(progress_val + "/" + myseekbar.getMax()); Toast.makeText(MainActivity.this,"SeekBar stopped", Toast.LENGTH_LONG).show(); } }); } }
Thats all! Thanks for reading!!
Stay tuned for further updates!!
Comments