Learn Android App Dev: Using Gestures
Hey there! This is Amey Lokhande. In this post, I'll be telling you on how to use Touch Gestures in your Android App. Using touch gestures you can make innovative apps. For ex, you can use gestures to change images, music or any kind of text. We will use the gestures such as Single or double tapping, on scroll and on flinging. These are some of the basic gestures pre-declared in the Android Studio.
So, here are the steps on how to do it:
1. First of all, drag a TextView in your layout. We will use this TextView to show which of the gesture is used. Thats all, for the layout. You can also use ImageView or VideoView for showing the gestures.
2. Now lets begin for the Java code. To make the app work properly import following classes.
import android.support.v4.view.GestureDetectorCompat; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.GestureDetector; import android.view.Menu; import android.view.MenuItem; import android.view.MotionEvent; import android.gesture.Gesture; import android.widget.TextView;
import static android.view.GestureDetector.*;
3. Now, let your MainActivity implement the OnGestureListener,OnDoubleTapListener interfaces. Now, to understand this your concepts of java must be clear. Well, what these interfaces will do is that they will allow the MainActivity class to use the pre-defined methods of it. So, your definition of MainActivity class will be like :
Also declare the variables for TextView and variable for GestureDetectorCompat in this MainActivity.

4. When you implement these interfaces, first you will get an error. But you need to rectify it by implementing it properly. Click on Implement Methods. And then you will get a Dialog Box.
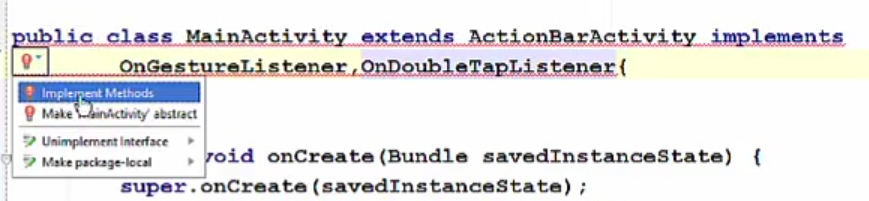
4. The dialog box which pops up shows you all the methods which can be possible gestures. So you just need to click OK when it pops.
5. So you will get all the Pre-Defined methods written by Android Studio in your code. Now, that you have got all the methods you just need to edit these Methods a little bit.

6. Now simply write this piece of code inside your OnCreate method. Here we have created an instance of the gesturedetect varibale.
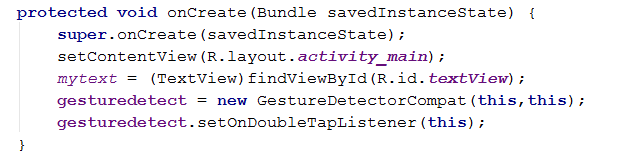
7. Now you need to do a simple step for printing which of the Gesture is used. Well, the syntax is simple as you know. Just you need to use textView.setText("Your Text")

7. Now the final step is to add an Override method 'onTouchEvent' which Android Studio will automatically generate for you.
All you need is to press 'ctrl+o' and then select the "onTouchEvent" method from it and you need to write this piece of code in it.

8. Now everything's done. You can run your App.
Java code:
package com.example.ameylokhande.gestureapp;
import android.support.v4.view.GestureDetectorCompat; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.GestureDetector; import android.view.Menu; import android.view.MenuItem; import android.view.MotionEvent; import android.gesture.Gesture; import android.widget.TextView;
import static android.view.GestureDetector.*;
public class MainActivity extends AppCompatActivity implements OnGestureListener,OnDoubleTapListener{ private static TextView mytext; private GestureDetectorCompat gesturedetect; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mytext = (TextView)findViewById(R.id.textView); gesturedetect = new GestureDetectorCompat(this,this); gesturedetect.setOnDoubleTapListener(this); }
@Override public boolean onTouchEvent(MotionEvent event) { gesturedetect.onTouchEvent(event); return super.onTouchEvent(event); }
@Override public boolean onSingleTapConfirmed(MotionEvent e) { mytext.setText("Single Tapped"); return false; }
@Override public boolean onDoubleTap(MotionEvent e) { mytext.setText("On double tap"); return false; }
@Override public boolean onDoubleTapEvent(MotionEvent e) { mytext.setText("On double tap event"); return false; }
@Override public boolean onDown(MotionEvent e) { mytext.setText("On down"); return false; }
@Override public void onShowPress(MotionEvent e) { mytext.setText("On show press"); }
@Override public boolean onSingleTapUp(MotionEvent e) { mytext.setText("On single tap up"); return false; }
@Override public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX, float distanceY) { mytext.setText("on scroll"); return false; }
@Override public void onLongPress(MotionEvent e) { mytext.setText("On long press"); }
@Override public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX, float velocityY) { mytext.setText("On Fling"); return false; } }
Well, this is it! Thanks for reading! Stay tuned for further updates!!