Designing patterns
Hey folks, today we are going to look at some interesting patters and how to design them using programming. In this article we will mainly concentrate about squares and triangles, we will try to construct the following figures :
1) Square :
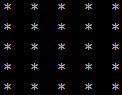
Consider the problem like a 2x2 matrix in which each element is "*"( just for representing) and printing all the elements, so that we can have a square filled with "*".
So we need 2 for loops for specifying rows and columns.
Code explanation:
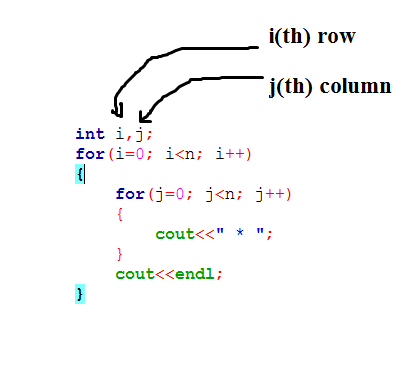
2) Hollow square
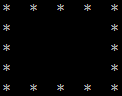
Now, just make some changes in the square code, we try to print white spaces in the range
0<p<n-1 and also 0<q<n-1.
Why?
Because this region corresponds to the cavity as shown
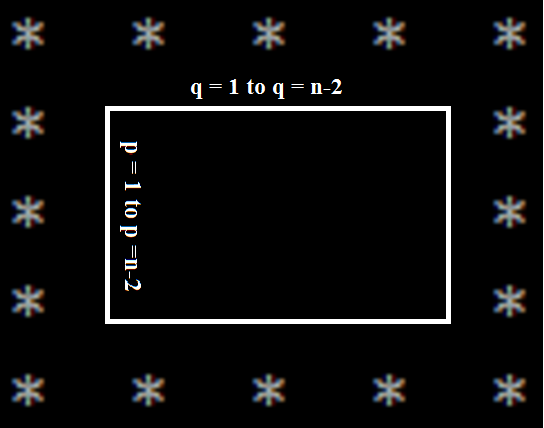
The same thing is implemented in the code :
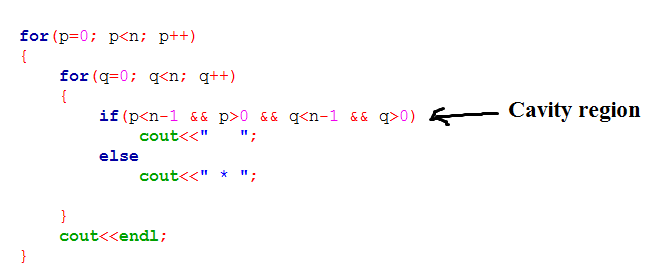
3) Hollow square with right diagonal :
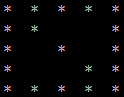
Think of it as a matrix with diagonal elements and we know that for diagonal elements the row and column is equal so instead of printing all white spaces in the previous code, print all white space except for the elements whose row and column no. is same.
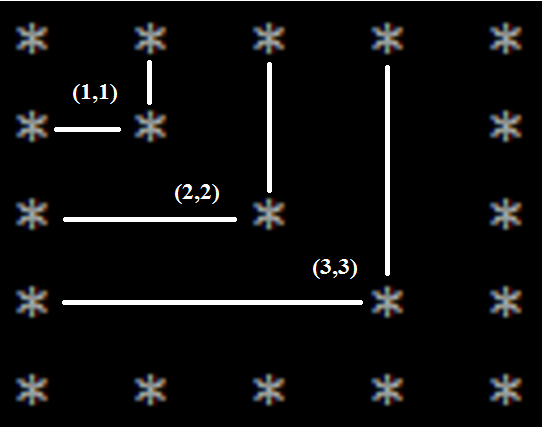
Hence, the code will look like this :
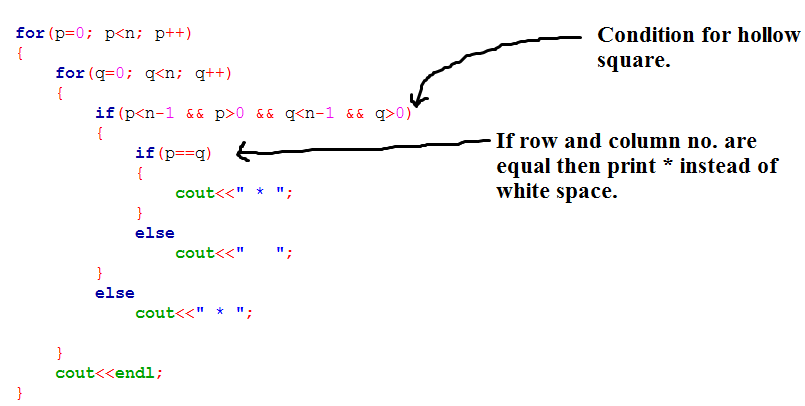
4) Hollow square with left diagonal :
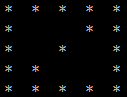
Here, now we can see that there is no pattern of equivalence of elements, but if we look in reverse like from bottom to up side we can observe the equivalence of row and column i.e if row and column no. have started from down side we would get diagonal elements on equating row and column value, to understand this see the figure below.
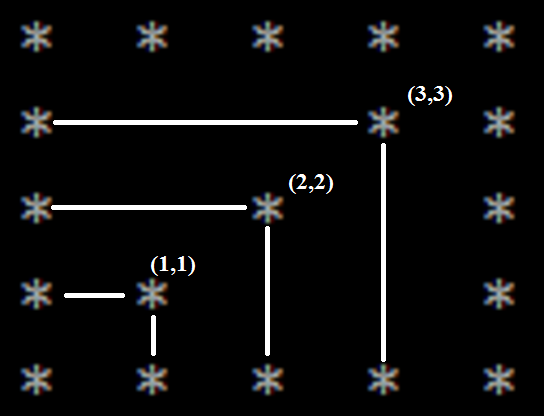
Now, in previous code we just need to do small modification in order to achieve this, while printing diagonal elements replace p with p+1 and q with n-q.
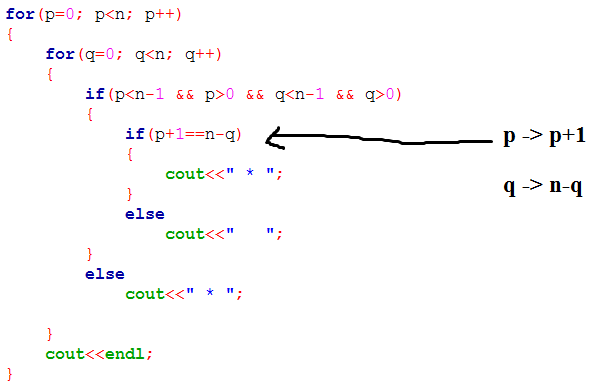
5) Hollow square with both the diagonals
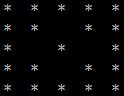
This case simply the OR of previous 2 cases, while printing diagonal take or of the conditions used previously in printing the diagonals.
Here's how the code will look :
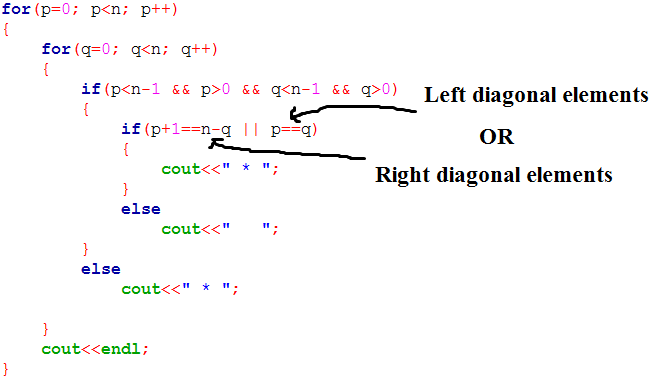
6) Triangle (Right-angled) :
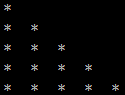
The concept is really simple just print the number of stars equal to the row number in which we are printing.
i.e 1st row has 1 star
2nd row have 2 stars.
3rd row have 3 stars....... and so on.
The code will look like this:
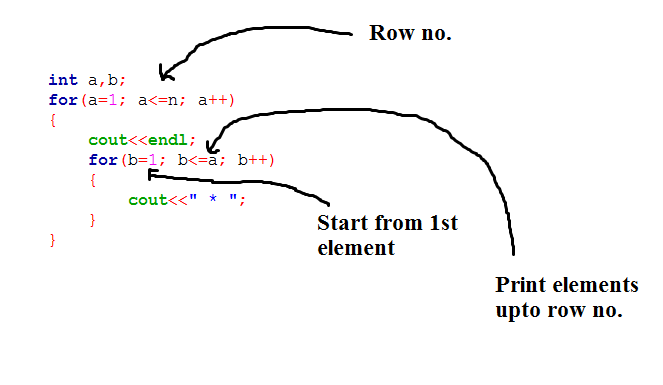
7) Right angled hollow triangle :
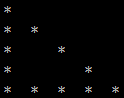
We already know how to construct a triangle with stars filled in it, extending the concept we can say that the hollow triangle will have only the starting and ending element for all the rows except for the last row which will obviously have all the elements and the same concept has been implemented in the code.
Code for the same :
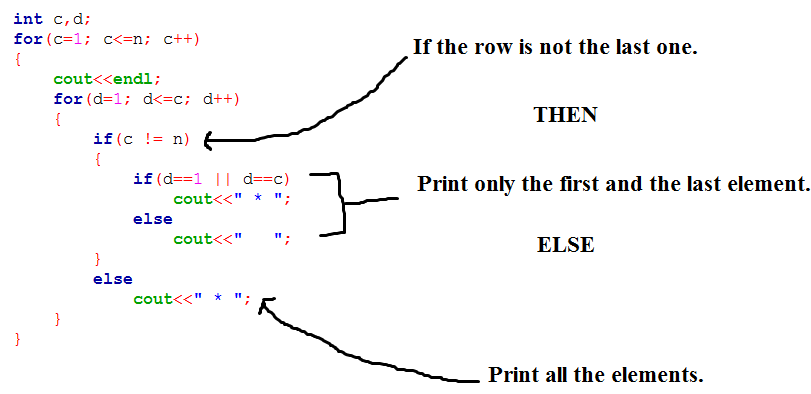
Note : You can create any kind of triangles with the code which I am going to explain next.
8) Pyramid shaped triangle (acute angled triangle)
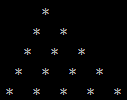
The concept I am going to tell you now can applied to create any type triangle with respect to angle i.e right angled, acute angled and obtuse angled.
At each row we need some specific amount of space before we start to print the first * of each row and that space is the row no. itself and we can adjust the amount of space to produce beautiful results.
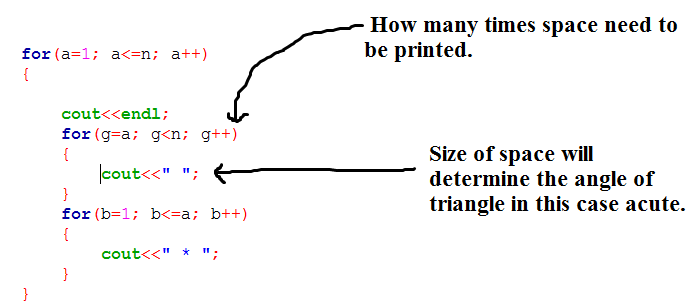
9) Hollow pyramid shaped triangle (Acute hollow triangle)
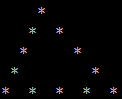
Just take the previous example and print only the last and first element for the all the rows except for the last one in which obviously have all the elements to be printed else the figure will not be a closed one.
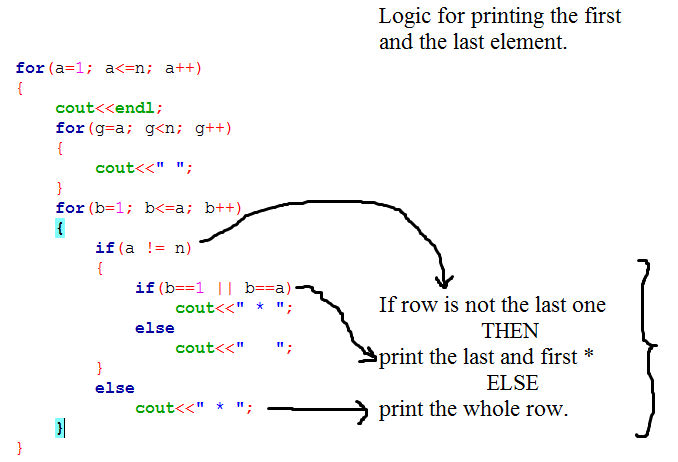
10) Right angled triangle with 90* angle on the left side (Normal + Hollow) using above geneal code :
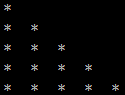
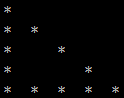
We just need to make the white space zero in the above code as there is no space when we start on a new row as shown in the code :
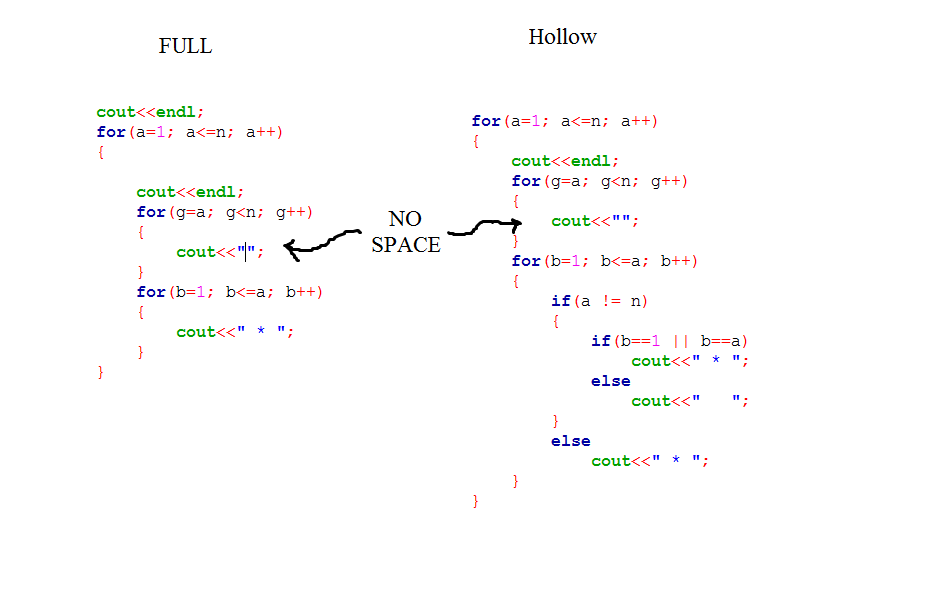
11) Right angled triangle with 90* angle on the right side (Normal + Hollow) using above geneal code :
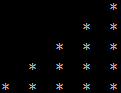
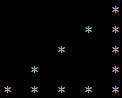
Put the amount of space as 3 units and the required pattern will be printed.
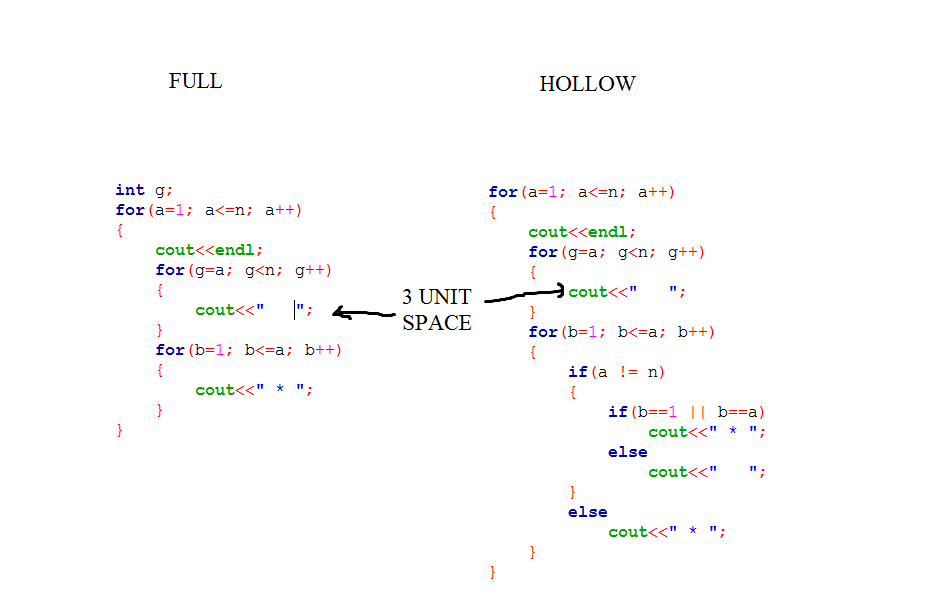
12) Obtuse angled triangle using general code (Hollow + Full)
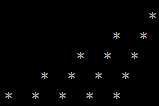
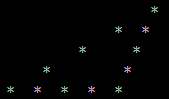
Use 4 units of spaces and you will get an obtuse angled triangle.
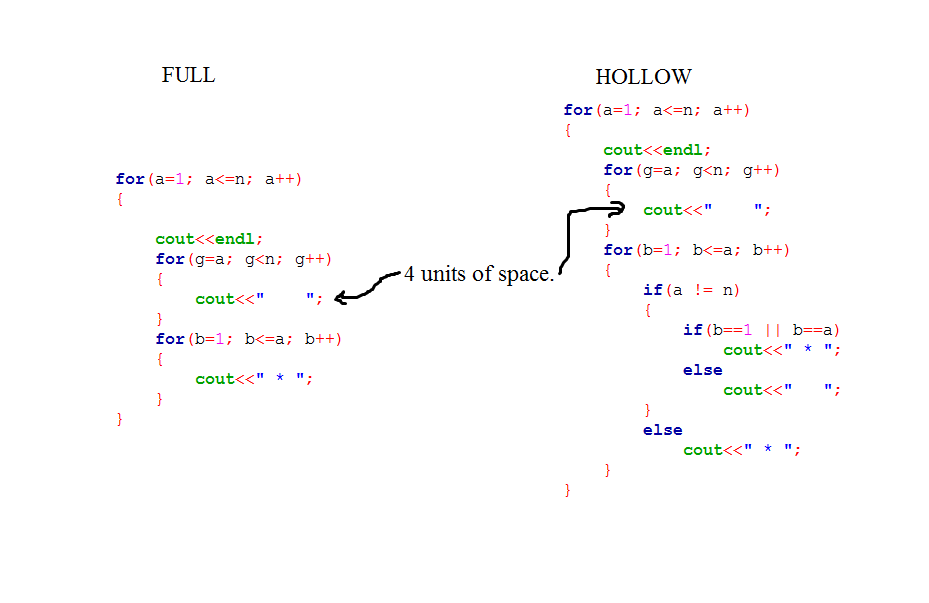
That's it for now guys, you must be wondering that why should you learn about patterns.
When we go for more complex problems about matrices then we must have prerequisite knowledge about how to access a particular pattern in a matrix, moreover pattern recognition is one of the important factors in fields like security encryption, Artificial Intelligence, Robotics and image processing, everything require pattern analysis, this is how real world problems are solved, there are patterns everywhere.
Full code in C++ for all the figures :
using namespace std;
int main() { int n; cout<<"Enter the value for n "; cin>>n; cout<<endl; //***** //***** //***** //***** //*****
int i,j; for(i=0; i<n; i++) { for(j=0; j<n; j++) { cout<<" * "; } cout<<endl; } cout<<endl; // **** // * * // * * // ****
int p,q; for(p=0; p<n; p++) { for(q=0; q<n; q++) { if(p<n-1 && p>0 && q<n-1 && q>0) cout<<" "; else cout<<" * ";
} cout<<endl; }
cout<<endl;
/* * * * * * * * * * * * * * * * * * * * */
for(p=0; p<n; p++) { for(q=0; q<n; q++) { if(p<n-1 && p>0 && q<n-1 && q>0) { if(p==q) { cout<<" * "; } else cout<<" "; } else cout<<" * ";
} cout<<endl; }
/* * * * * * * * * * * * * * * * * * * * */
cout<<endl; for(p=0; p<n; p++) { for(q=0; q<n; q++) { if(p<n-1 && p>0 && q<n-1 && q>0) { if(p+1==n-q) { cout<<" * "; } else cout<<" "; } else cout<<" * ";
} cout<<endl; }
/* * * * * * * * * * * * * * * * * * * * * * */
cout<<endl; for(p=0; p<n; p++) { for(q=0; q<n; q++) { if(p<n-1 && p>0 && q<n-1 && q>0) { if(p+1==n-q || p==q) { cout<<" * "; } else cout<<" "; } else cout<<" * ";
} cout<<endl; }
/* * * * * * * * * * * * * * * * */
int a,b; for(a=1; a<=n; a++) { cout<<endl; for(b=1; b<=a; b++) { cout<<" * "; } }
/* * * * * * * * * * * * * */
cout<<endl<<endl; int c,d; for(c=1; c<=n; c++) { cout<<endl; for(d=1; d<=c; d++) { if(c != n) { if(d==1 || d==c) cout<<" * "; else cout<<" "; } else cout<<" * "; } }
/* * * * * * * * * * * * * * * * */
cout<<endl; int g; for(a=1; a<=n; a++) { cout<<endl; for(g=a; g<n; g++) { cout<<" "; } for(b=1; b<=a; b++) { cout<<" * "; } }
cout<<endl<<endl;
/* * * * * * * * * * * * * */ for(a=1; a<=n; a++) { cout<<endl; for(g=a; g<n; g++) { cout<<" "; } for(b=1; b<=a; b++) { if(a != n) { if(b==1 || b==a) cout<<" * "; else cout<<" "; } else cout<<" * "; } }
/* * * * * * * * * * * * * * * * */
cout<<endl; for(a=1; a<=n; a++) {
cout<<endl; for(g=a; g<n; g++) { cout<<""; } for(b=1; b<=a; b++) { cout<<" * "; } }
/* * * * * * * * * * * * * */
cout<<endl; for(a=1; a<=n; a++) { cout<<endl; for(g=a; g<n; g++) { cout<<""; } for(b=1; b<=a; b++) { if(a != n) { if(b==1 || b==a) cout<<" * "; else cout<<" "; } else cout<<" * "; } } /* * * * * * * * * * * * * * * * */
cout<<endl; for(a=1; a<=n; a++) {
cout<<endl; for(g=a; g<n; g++) { cout<<" "; } for(b=1; b<=a; b++) { cout<<" * "; } } /* * * * * * * * * * * * * */
cout<<endl; for(a=1; a<=n; a++) { cout<<endl; for(g=a; g<n; g++) { cout<<" "; } for(b=1; b<=a; b++) { if(a != n) { if(b==1 || b==a) cout<<" * "; else cout<<" "; } else cout<<" * "; } }
return 0;
}
Sample INPUT/OUTPUT :
n = 5
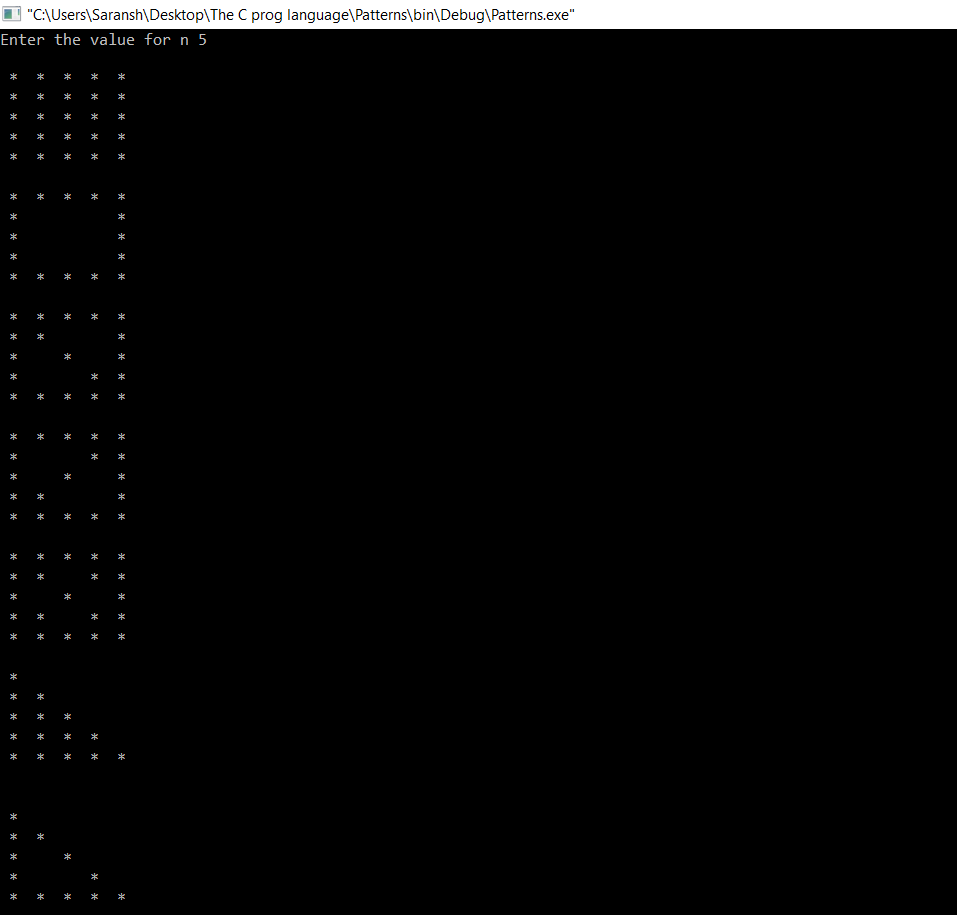
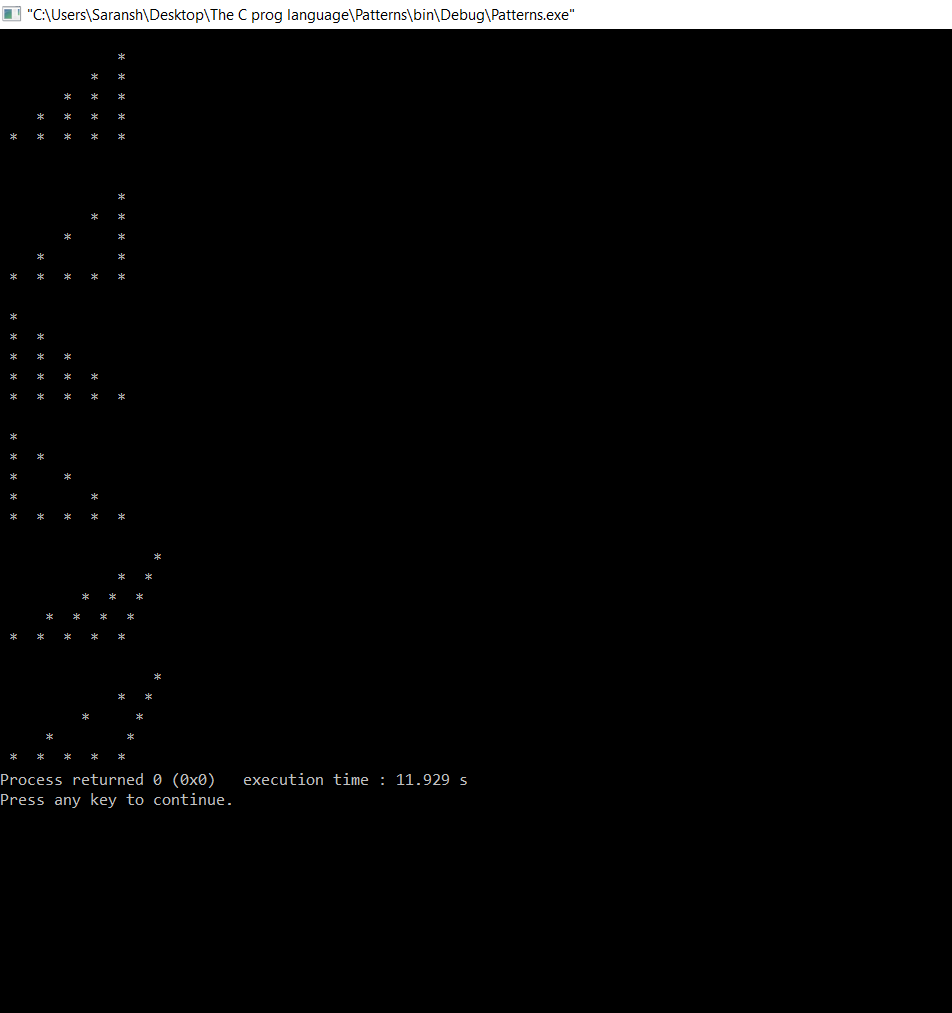
n = 20:
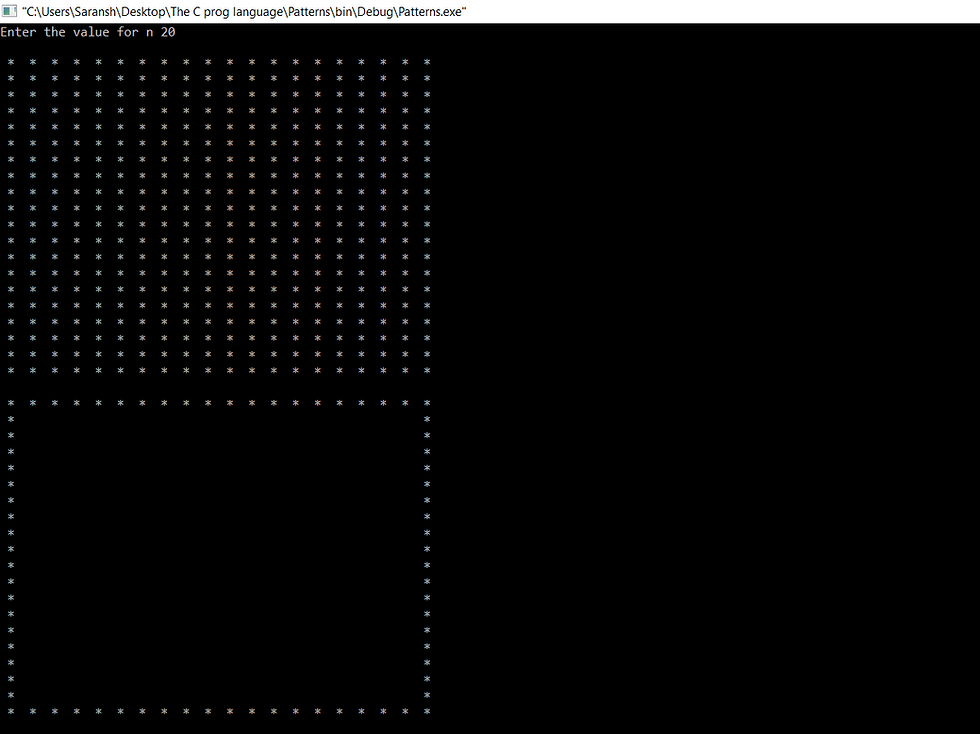
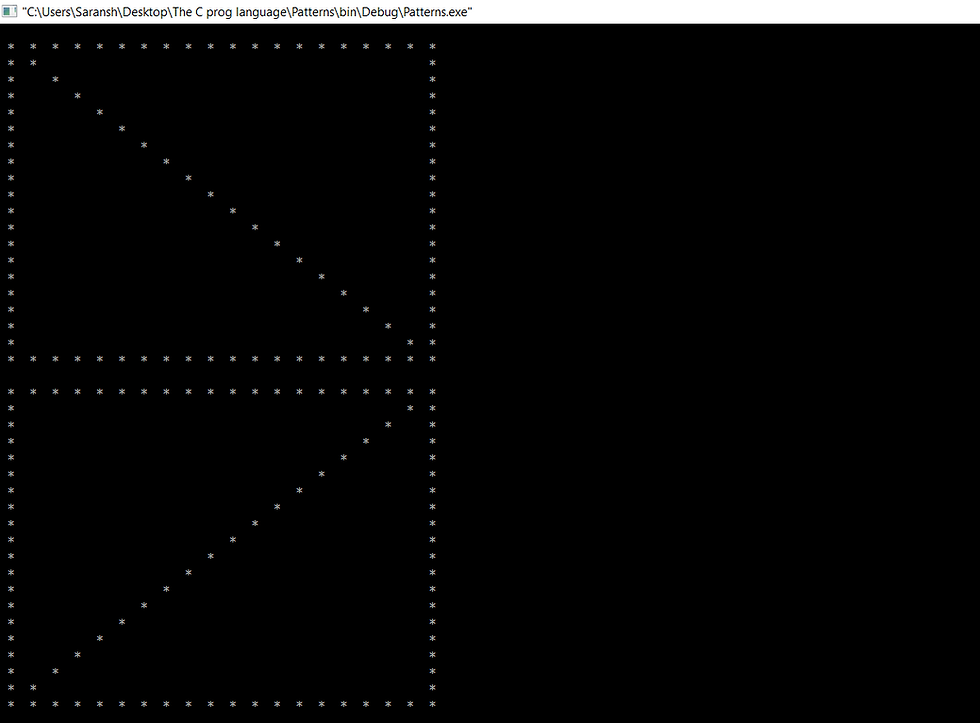
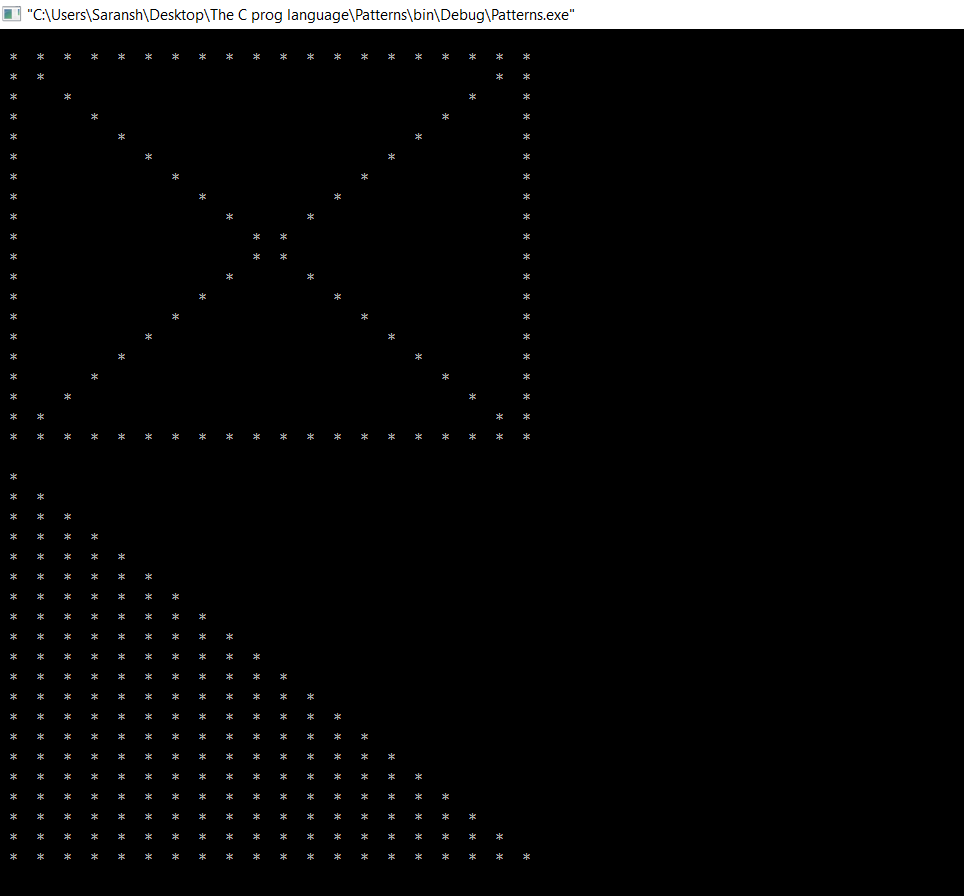
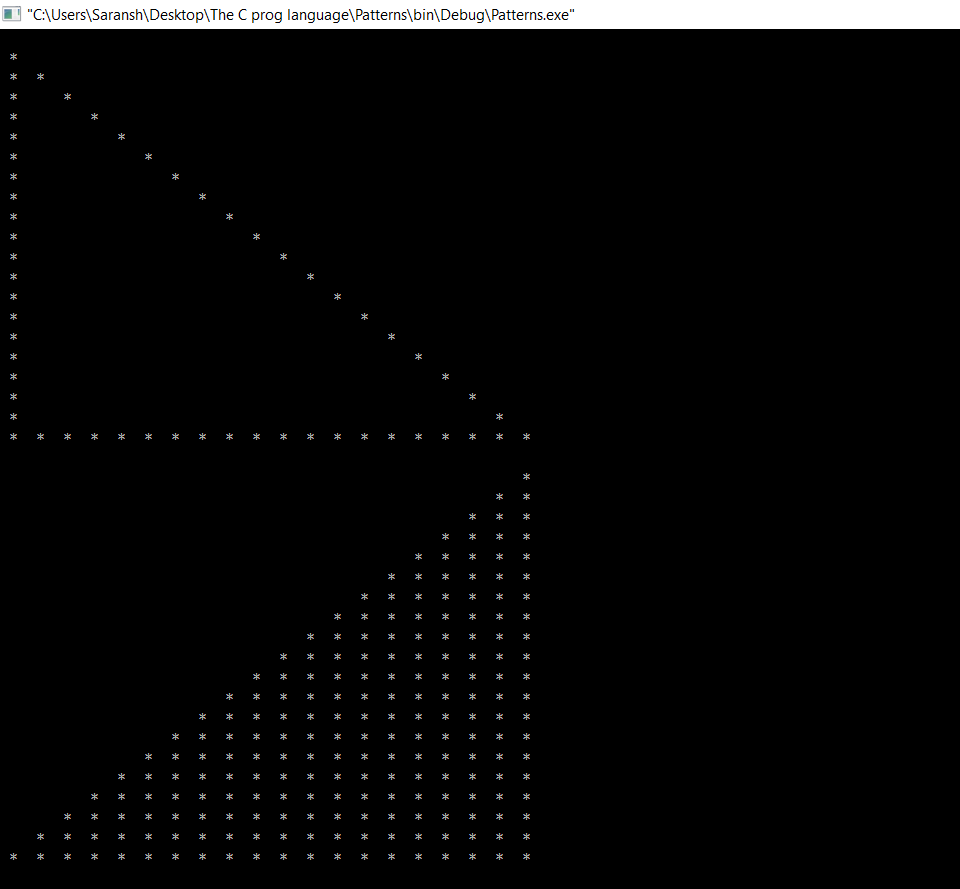
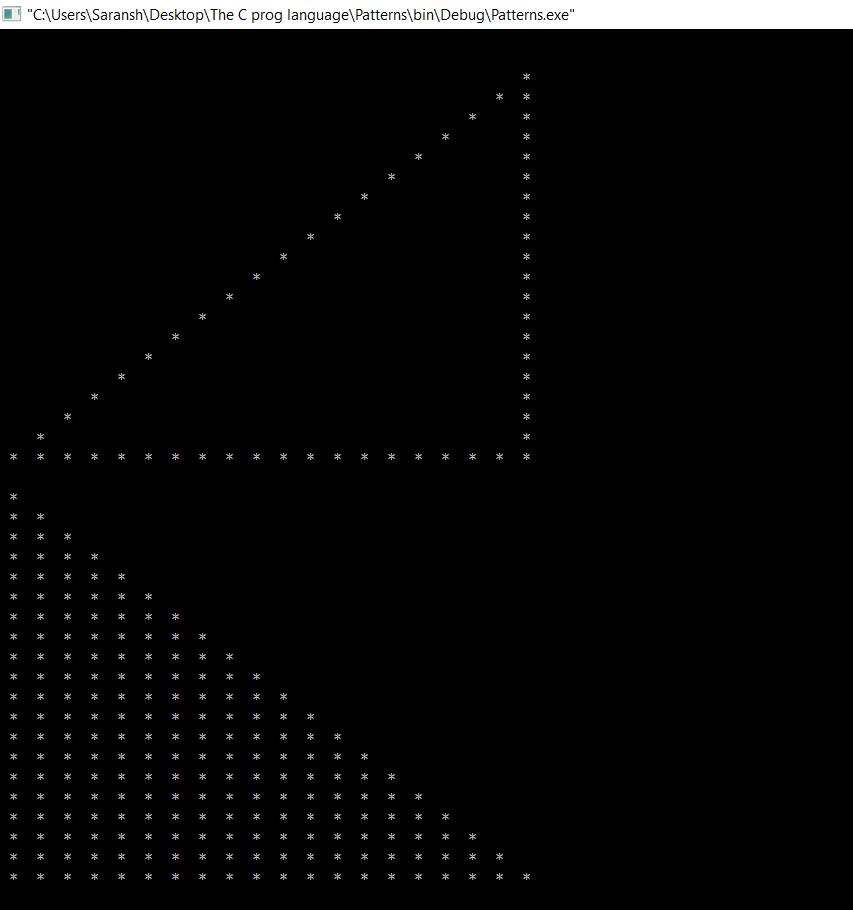
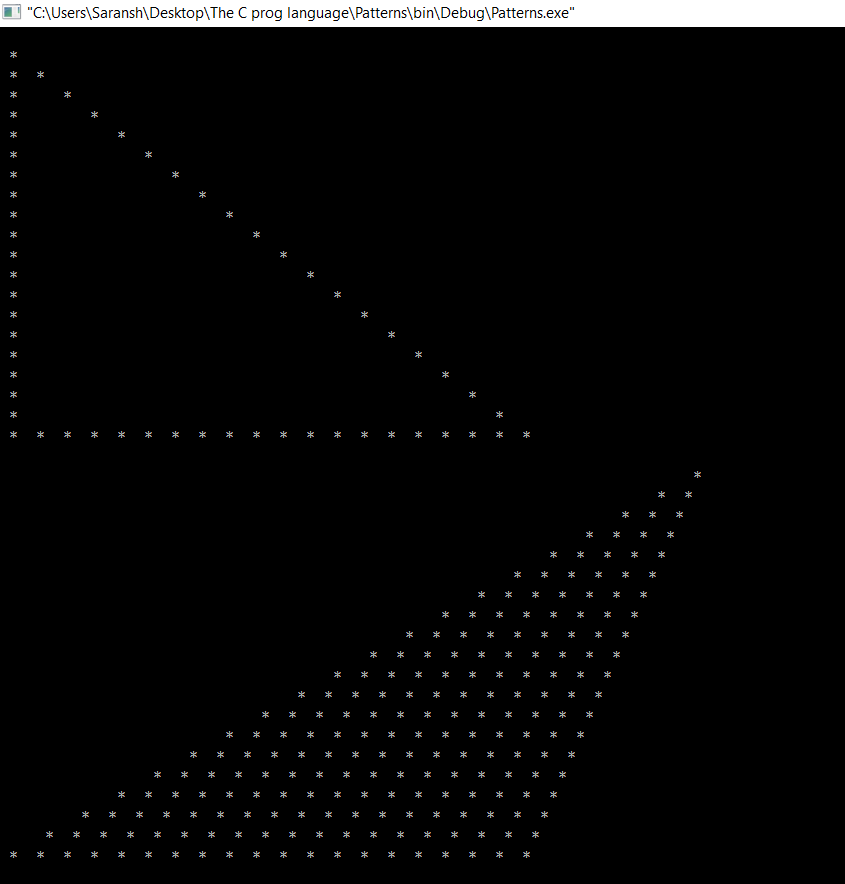
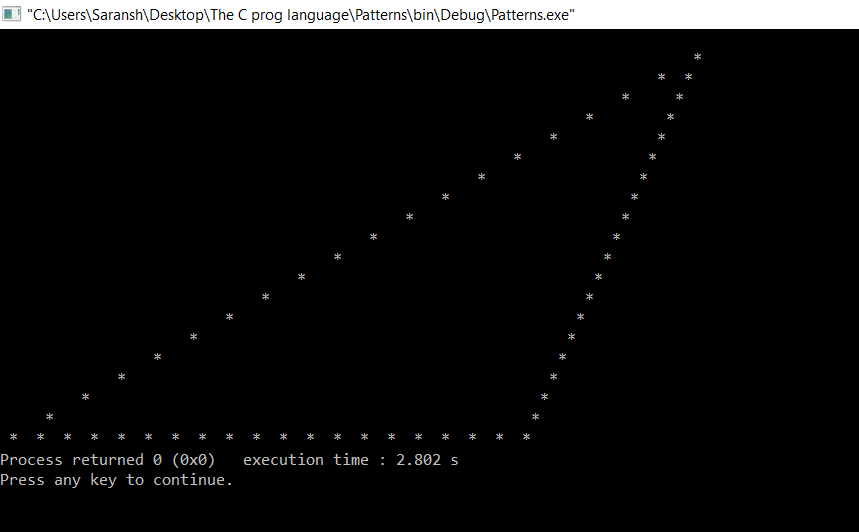