Taking Input from the user and Math operation
- Mayank Agarwal
- Jan 19, 2017
- 2 min read
Hi guys, this is Mayank Agarwal today we’ll see how to take input from the user in Java programing language and then we create a small program that will work as a calculator.
To take a input from the user there is a special class already define in the Java programming language i.e. “Scanner”.
To use Scanner in your program you’ll need to include a header file.
“import java.util.Scanner;”.
The syntax for Scanner class is
Scanner instance = new Scanner(System.in);
datatype variable_name = instance.nextInt for integer / instance.nextLine for string / instance.nextDouble for double etc.
Don’t worry we’ll see this thing again through an example.
In the figure shown below, we are taking an integer number as an input from the user.
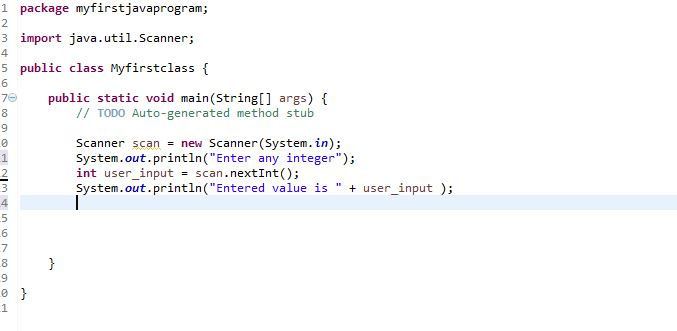
Here scan is the instance and user_input is the variable name. There is one more thing that I used in this program which is called concatenation. You can see that there is a plus sign in the System.out line what it will do is like it will concatenate "Enter value is " string and the value of user_input. Suppose the entered value is 14 then the String that will be display on the system will be "Entered value is 14" in a single line.
What scan.nextInt() will do is like it tell the java that we are going to input an integer as an input.( You will see that as soon as you'll write scan. there will be a lot of option will start displaying on your screen.
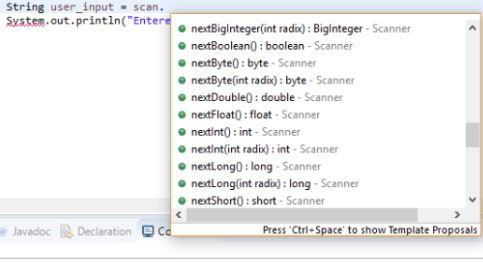
Now to take other types of input from the user you'll just need to make couple of changes
1. Change the datatype of the variable.
2. Instead of using scan.nextInt you have to used scan.nextLine() for inputting a string or scan.nextDouble() for double etc.
The picture shown some example for inputting a string and a float.

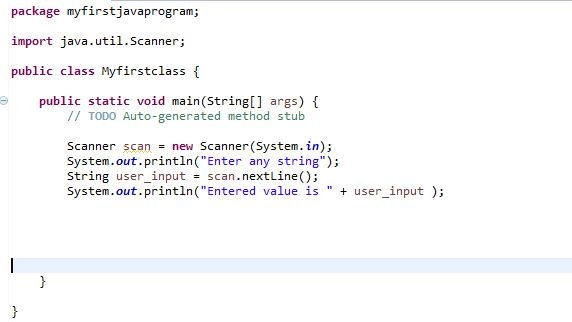
Now we are going to learn about math operation in java programming language through a small program.
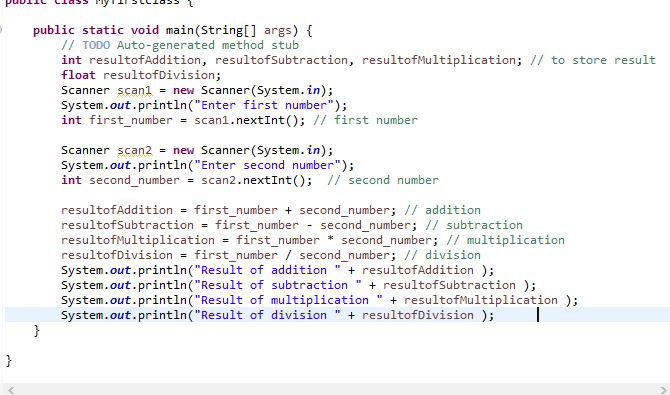
Output
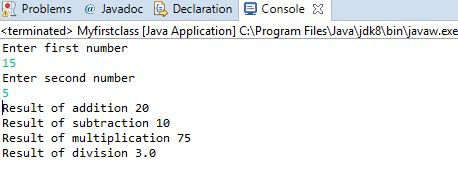
Now let's understand the code line by line,

Declaration of variable to store the results. Note: We have used datatype float for storing the result of division because the division of two might gives the result in decimal.

To take an integer from the user. Here scan1 is the instance or you can give any other name of your choice it really doesn't matter.

For addition you just need to use "+" operator. Similarly for Subtraction we need to use "-" operator. Same for the case of multiplication we uses "*" and division "/".
That's it for this tutorial.
Comments