2D array sort
- Saransh Dabas
- Jan 15, 2017
- 2 min read
Problem Statement:
You are given an arbitrary n*m matrix and you have to sort all the elements in ascending order.
For example :
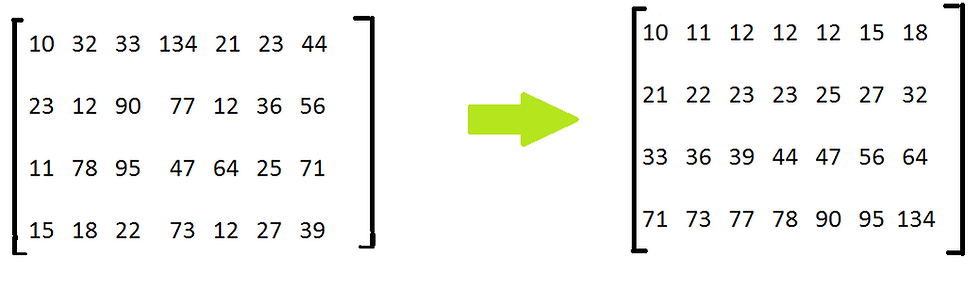
You can see the output in Sample INPUT/OUTPUT, at the end of the post.
Now we can solve this problem very simply, I will tell you what I thought when I first heard this problem from my friend. I thought that why even consider this problem complicated, means sorting the elements by migrating through each row will be difficult or we can say that sorting of individual rows can be done easily but what about sorting among rows?
We are already familiar with sorting of single dimensional array so just take all the elements from the 2D array and put it in 1D array then sort it and then put them back in the original 2D array.
Note: The idea can be extended to n-dimensional array.
Elements of 2D array --------> Elements to 1D array
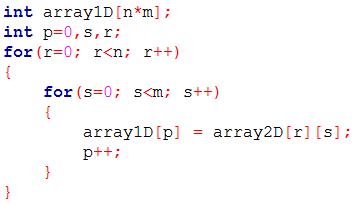
Sorting method (Quick Sort)
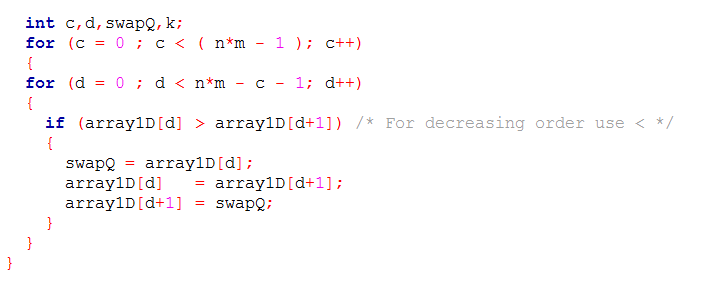
Write Back of sorted elements

Full C++ implementation/Code:
int main() { int n,m; cout<<"Enter the no. of rows"<<endl; cin>>n; cout<<"Enter the no. of columns"<<endl; cin>>m; int array2D[n][m]; int i,j; for(i=0; i<n; i++) { for(j=0; j<m; j++) { cout<<"Enter the "<<i<<j<<" element of array "; cin>>array2D[i][j];
} }
int array1D[n*m]; int p=0,s,r; for(r=0; r<n; r++) { for(s=0; s<m; s++) { array1D[p] = array2D[r][s]; p++; } }
int c,d,swapQ,k; for (c = 0 ; c < ( n*m - 1 ); c++) { for (d = 0 ; d < n*m - c - 1; d++) { if (array1D[d] > array1D[d+1]) /* For decreasing order use < */ { swapQ = array1D[d]; array1D[d] = array1D[d+1]; array1D[d+1] = swapQ; } } }
int f1, f2,a = 0; for(f1=0; f1<n; f1++) { for(f2=0; f2<m; f2++) { array2D[f1][f2] = array1D[a]; a++; } } cout<<endl; cout<<"The sorted 2D array is"<<endl; int d1,d2; for(d1=0; d1<n; d1++) { for(d2=0; d2<m; d2++) { cout<<array2D[d1][d2]; cout<<" ";
} cout<<endl; }
return 0; }
Sample INPUT/OUTPUT :
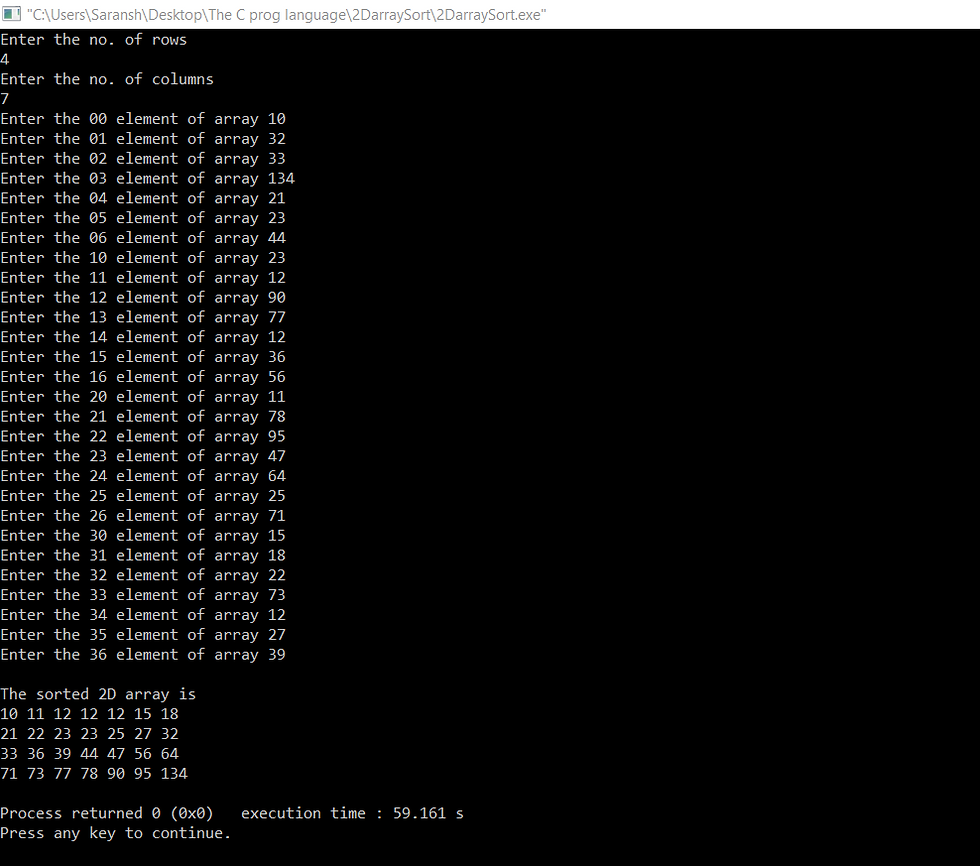
Thanks for reading guys. Happy coding!