Matrix-Multiplication
In mathematics, matrix multiplication or the matrix product is a binary operation that produces a matrix from two matrices.
Let us consider two matrices A and B.
The product of two matrices AB is defined only if the number of columns in A is equal to the number of rows in B.
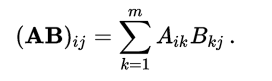
This is the formal definition of matrix multiplication, we will try to understand with an example.
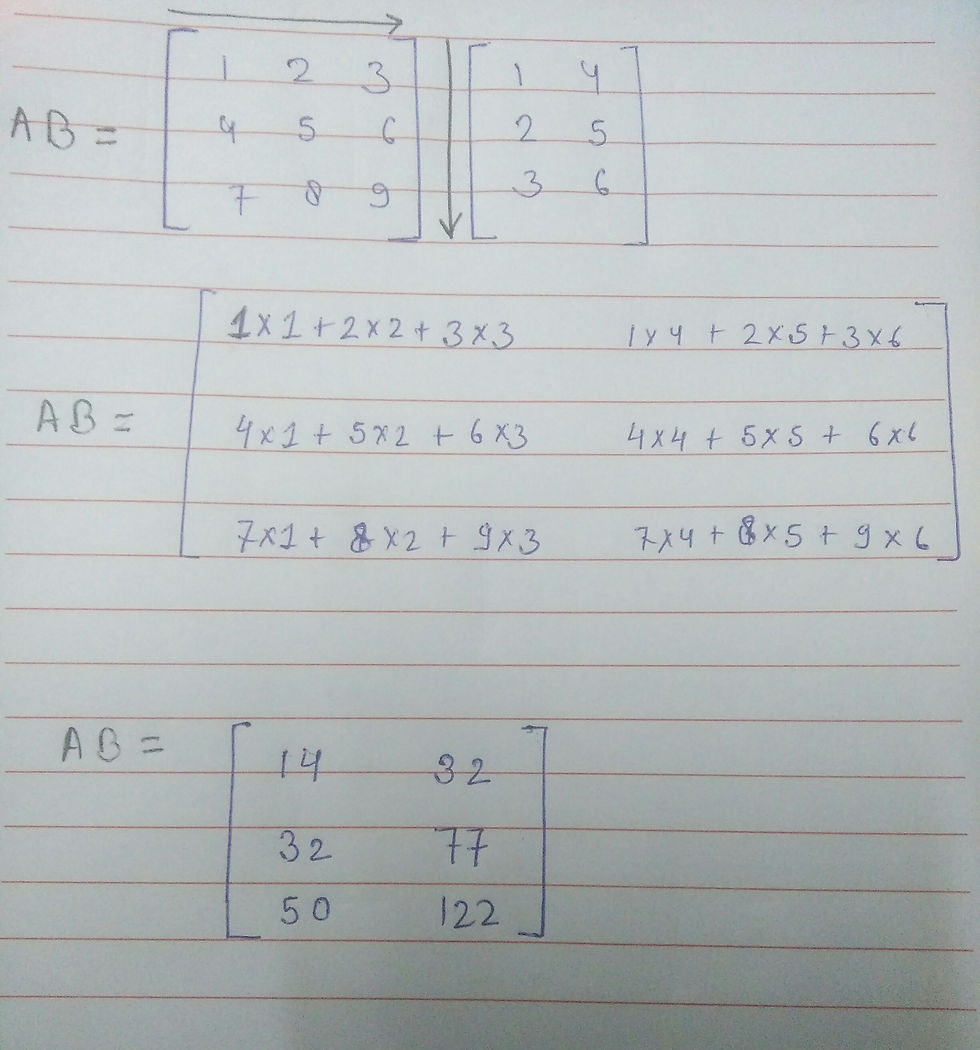
Some examples in terms of variables :
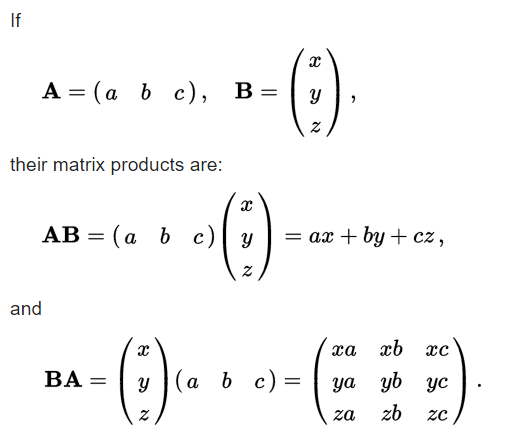
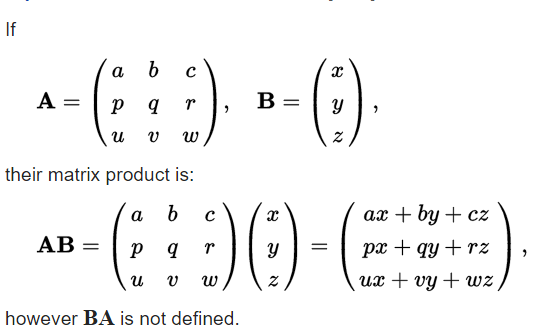
Now how to solve this problem using programming!
See my main aim is not to fed you guys up with formal definitions, algorithms and pseducodes etc., there are already plenty of sites doing a great job, instead I will help you with how to think for solving a problem and then we will implement our thinking in c++ programming, there is no need of object oriented programming as for now.
Let us suppose the dimensions of 2 matrices are n1*m1 and n2*m2 respectively, now it is clear that the resultant matrix will have dimensions n1*m2, so we need two for loops which will define coordinate of each element of resultant matrix.
Outer for loop will decide row coordinate and Inner for loop will take care of column coordinate. Now we have the required matrix framework ready , now the best part is to fill this dumb framework.
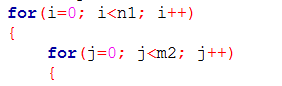
Filling of the elements
Now inside the inner for loop we will need another for loop which will loop as many times as the number of columns of the first matrix or the number of rows in second matrix.
Along with this a temporary variable will take care of subsequent row to column mapped multiplication with addition.
The key point is row to column mapping, so what will we do, we will make use of three variables
i (outer for loop)
j (inner for loop)
p (inside inner for loop)
i will take care that each row in 1st matrix participate in multiplication
j will take care that each column in 2nd matrix participate in multiplication
p's significance for 1st matrix is to iterate through elements of each row
p's significance for 1st matrix is to iterate through elements of each column
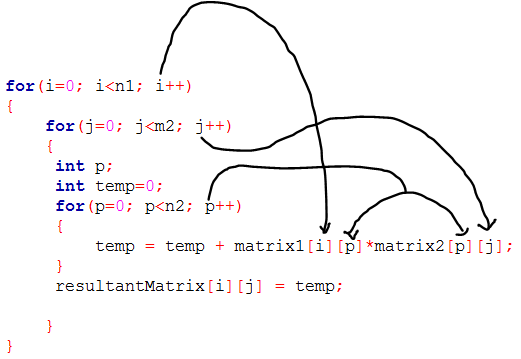
Check this figure out, it will be more clear;
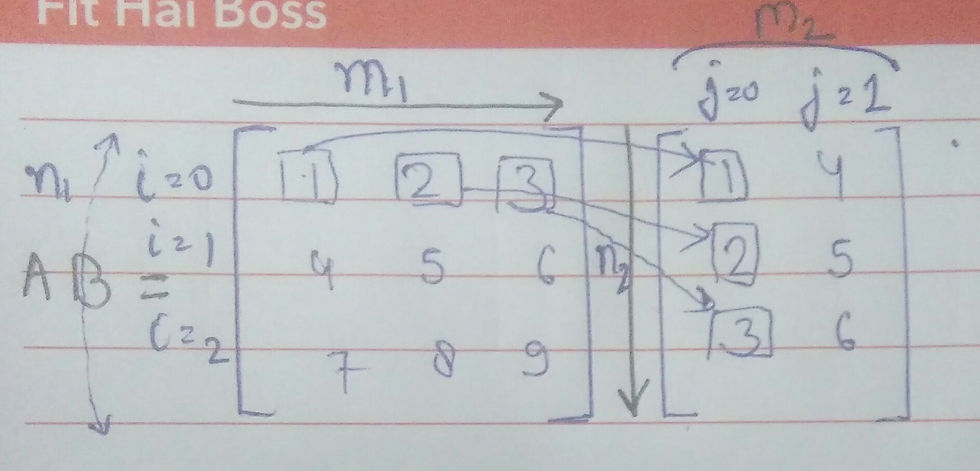
Now, at this point I will recommend to try it out yourself, complete implementation is given below:
C++ implementation :
int main() { int n1; int m1; int n2; int m2;
// Taking inputs for rows and columns
cout<<"Enter the no. of rows in 1st matrix "; cin>>n1; cout<<"Enter the no. of columns in 1st matrix "; cin>>m1; cout<<"Enter the no. of rows in 2nd matrix "; cin>>n2; cout<<"Enter the no. of columns in 2nd matrix "; cin>>m2;
if(m1 == n2) // Checking whether the matrices are multiplicable or not. { int matrix1[n1][m1]; int matrix2[n2][m2]; int a,b,c,d;
for(a=0; a<n1; a++) // Taking inputs for elements in 1st matrix { for(b=0; b<m1; b++) { cout<<"Enter the "<<a<<b<<" element of 1st matrix"<<endl; cin>>matrix1[a][b]; } }
for(c=0; c<n2; c++) // Taking inputs for elements in 1st matrix { for(d=0; d<m2; d++) { cout<<"Enter the "<<c<<d<<" element of 2nd matrix"<<endl; cin>>matrix2[c][d]; } }
// Actual Multiplication starts here int resultantMatrix[n1][m2]; int i,j; int tempElement; for(i=0; i<n1; i++) { for(j=0; j<m2; j++) { int p; int temp=0; for(p=0; p<n2; p++) { temp = temp + matrix1[i][p]*matrix2[p][j]; } resultantMatrix[i][j] = temp;
} } int x,y; cout<<"The resultant matrix is"<<endl; for(x=0; x<n1; x++) // Displaying the resultant matrix { for(y=0; y<m2; y++) { cout<<resultantMatrix[x][y]<<" "; } cout<<endl; } } else { cout<<"The no. of columns in the first matrix is not equal to the no. of rows of second matrix."; } }